Here’s how it looks in basic CSS code:
CSS
p { /* Targets all paragraph elements */
color: red;
}
In this example, all paragraph text within your HTML would be changed to red. Of course, red is just the beginning! There are a multitude of ways to express color values in CSS, providing you with a vast palette with which to experiment.
Color Values: Your Textual Rainbow
Color Names
CSS offers a collection of basic color names for the most common colors. Think “red,” “blue,” “yellow,” “green,” and so on. These are straightforward to remember, making them an excellent starting point for beginners. Here’s an example:
CSS
h1 {
color: orange;
}
The main advantage of color names is their simplicity. However, you’re limited to a relatively small set of colors, which may only sometimes match your precise design vision.
Grow Your Sales
- Incredibly Fast Store
- Sales Optimization
- Enterprise-Grade Security
- 24/7 Expert Service
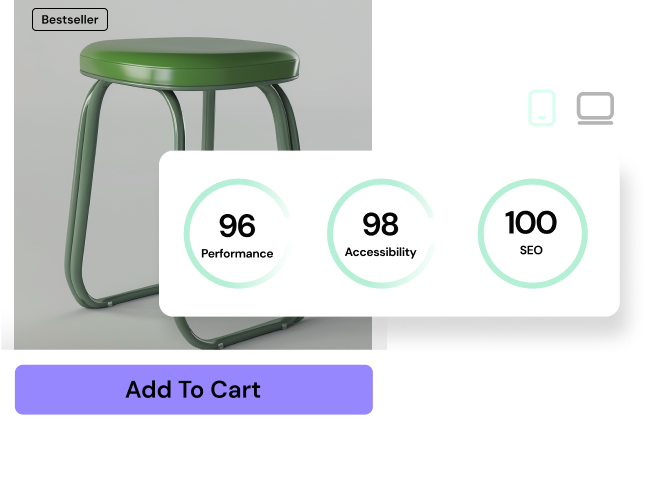
- Incredibly Fast Store
- Sales Optimization
- Enterprise-Grade Security
- 24/7 Expert Service
- Prompt your Code & Add Custom Code, HTML, or CSS with ease
- Generate or edit with AI for Tailored Images
- Use Copilot for predictive stylized container layouts

- Prompt your Code & Add Custom Code, HTML, or CSS with ease
- Generate or edit with AI for Tailored Images
- Use Copilot for predictive stylized container layouts
- Craft or Translate Content at Lightning Speed
Top-Performing Website
- Super-Fast Websites
- Enterprise-Grade Security
- Any Site, Every Business
- 24/7 Expert Service

Top-Performing Website
- Super-Fast Websites
- Enterprise-Grade Security
- Any Site, Every Business
- 24/7 Expert Service
- Drag & Drop Website Builder, No Code Required
- Over 100 Widgets, for Every Purpose
- Professional Design Features for Pixel Perfect Design
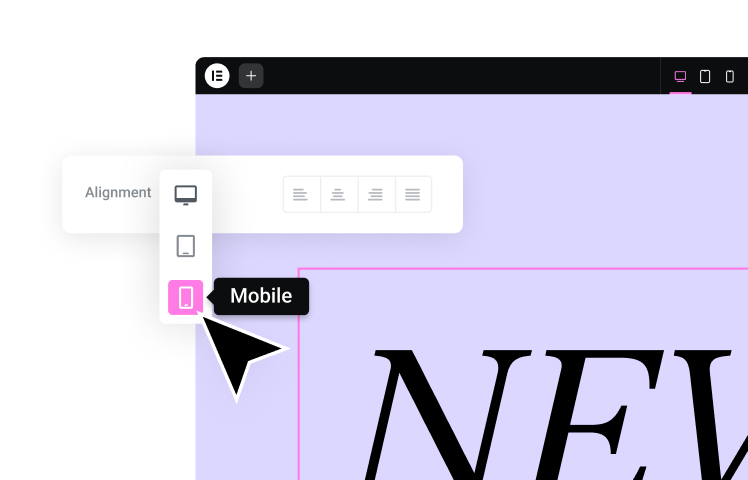
- Drag & Drop Website Builder, No Code Required
- Over 100 Widgets, for Every Purpose
- Professional Design Features for Pixel Perfect Design
- Marketing & eCommerce Features to Increase Conversion
- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking

- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking
Hexadecimal Color Codes
Hexadecimal color codes, starting with a “#”, provide a vastly expanded color palette. They consist of six characters representing the Red, Green, and Blue (RGB) components of a color. For example, #FF0000 is pure red, while #008000 is a deep green.
CSS
p {
color: #F26522; /* A warm orange tone */
}
Hex codes grant you precision and millions of color possibilities. Many design tools and websites offer color pickers that output hex codes, making it easy to find the exact shade you want. The only slight downside is that they can be less intuitive to read at first glance compared to color names.
RGB and RGBA Colors
RGB offers yet another way to define colors based on their Red, Green, and Blue components. Within the rgb() function, you specify the intensity of each component on a scale of 0 to 255. For instance, rgb(255, 0, 0) produces the same pure red as #FF0000.
CSS
h2 {
color: rgb(128, 0, 128); /* A rich purple */
}
The true power of RGB comes with the addition of an alpha channel, giving you RGBA. This ‘A’ stands for “alpha” and controls transparency. RGBA values are expressed as rgba(R, G, B, A), where the alpha value ranges from 0 (fully transparent) to 1 (fully opaque). Here’s how to create a semi-transparent text overlay:
CSS
.overlay-text {
color: rgba(0, 0, 0, 0.6); /* Black text with 60% opacity */
}
RGBA is ideal for layering text over images or backgrounds, giving you fine-grained control over how much the underlying elements show through.
HSL and HSLA Colors
HSL (Hue, Saturation, Lightness) is a color model that aligns more closely with the way humans perceive color. It’s expressed as hsl(H, S, L):
- Hue is the type of color on a 360-degree color wheel (0 is red, 120 is green, 240 is blue, etc.).
- Saturation: The intensity of the color (0% is gray, 100% is full color)
- Lightness: How bright or dark the color is (0% is black, 100% is white)
HSLA simply adds an alpha channel for transparency, just like RGBA.
CSS
p {
color: hsl(30, 100%, 50%); /* A vibrant orange */
}
HSL can sometimes be more intuitive for beginners to grasp. It allows you to think in terms of color types and vibrancy rather than mixing individual red, green, and blue components.
Methods for Changing Text Color
There are three primary methods to change text color using CSS. Each technique offers different levels of control and specificity, allowing you to target the elements you want to style precisely.
Inline Styles
Inline styles are applied directly within an HTML element’s opening tag using the style attribute. Let’s change the color of a specific paragraph:
HTML
<p style="color: blue;">This paragraph will have blue text.</p>
Inline styles are handy for quick, one-off changes to individual elements. However, they have some drawbacks:
- Clutter: They make your HTML harder to read and maintain.
- Specificity: Inline styles will override other, more general CSS rules, sometimes leading to unexpected styling issues.
Therefore, it’s usually best to use inline styles sparingly and only when absolutely necessary.
Internal Stylesheets
Internal stylesheets use <style> tags placed within the <head> section of your HTML document. This allows you to define styles for specific HTML elements:
HTML
<head>
<style>
h1 {
color: #009933; /* A dark green */
}
p {
color: #333333; /* A dark gray */
}
</style>
</head>
Internal stylesheets are excellent for styling an entire webpage. They offer these advantages:
- Centralization: Keeps your styling rules in one place within the HTML.
- Specificity: Internal styles take precedence over most browser default styles but are less specific than inline styles.
External Stylesheets
External stylesheets are the recommended approach for managing your website’s CSS. You create a separate .css file and link it to your HTML document using the <link> tag within the <head> section:
HTML
<head>
<link rel="stylesheet" href="styles.css">
</head>
styles.css:
CSS
body {
color: #222222; /* Sets default text color for the page */
}
h1 {
color: #CD5C5C; /* Indian red for headings */
}
a {
color: #0000EE; /* Standard blue for links */
}
External stylesheets provide several key benefits:
- Maintainability: Separating CSS from HTML keeps your code organized and easier to update.
- Reusability: A single stylesheet can be applied to multiple HTML pages, ensuring a consistent look across your website.
- Preprocessors: You can use advanced CSS preprocessors like Sass or Less to streamline your stylesheet development (we’ll touch on this in a future article!).
CSS Specificity and Inheritance
Understanding CSS specificity and inheritance is important to avoid unexpected text color changes. Specificity refers to how CSS determines which rule takes precedence when multiple conflicting styles for an element exist. Generally, more specific selectors (like IDs) will override less specific ones (like HTML tags).
Inheritance means that some CSS properties, like color, are passed down from parent elements to their child elements. This means if you set a text color on the <body> tag, all text within the page will inherit that color unless overridden by more specific styles.
Targeting Specific Elements
CSS gives you fine-grained control over which text elements to style. Let’s look at the most common targeting methods:
HTML Tags
The simplest way is to target generic HTML tags. This will apply your color change to all elements of that type within your page:
CSS
p {
color: #9932CC; /* Dark orchid for paragraphs */
}
Classes
Classes provide a more flexible way to apply text color styles. You define a class in your CSS and then add the class attribute to the HTML elements you want to target:
CSS
.highlight {
color: #FFD700; /* A vibrant gold */
}
HTML
<p class="highlight">This text will be highlighted in gold.</p>
Classes are excellent because:
- Reusability: You can apply the same class to multiple elements, ensuring consistent styling.
- Organization: Classes help structure your CSS and make it easier to understand.
IDs
IDs are unique identifiers for specific HTML elements and use the id attribute. Use IDs sparingly, as they have a very high specificity that can override other styles.
CSS
#important-message {
color: red;
}
HTML
<p id="important-message">This message will have red text.</p>
IDs are primarily useful for styling single, distinct elements and often find use in JavaScript interactions.
Changing Text Color on User Interactions
Hover Effects
The :hover pseudo-class adds a special touch to your website, letting you change text color when a user hovers their mouse over an element. This is a common technique for highlighting links or buttons:
CSS
a:hover {
color: #EE82EE; /* A playful violet for link hover */
}
.button:hover {
color: white; /* White text on button hover */
}
Hover effects provide visual feedback, indicating to the user that an element is interactive. They enhance navigation and can make your website feel more engaging.
Other States
CSS offers additional pseudo-classes to style text based on different interaction states:
- :active: When an element is being clicked or tapped.
- :visited: For styling links, the user has already visited.
- :focus: When an element (like a form field) has keyboard focus.
Let’s make visited links a less saturated color:
CSS
a:visited {
color: #800080; /* A muted purple for visited links */
}
These pseudo-classes give you even more control over how your text responds to user actions, further improving the user experience.
Accessibility and Color Contrast
The Importance of Sufficient Contrast
Choosing text and background colors with sufficient contrast is essential to make your website readable for everyone, including users with visual impairments. The Web Content Accessibility Guidelines (WCAG) define minimum contrast ratios to ensure your content is legible.
Poor color contrast can make text difficult or even impossible to read, leading to frustration and exclusion for some users. By prioritizing contrast, you create a more welcoming and inclusive online experience.
Color Blindness Simulators
Color blindness affects a significant portion of the population. Color blindness simulators are valuable tools that help you visualize how your website appears to people with different types of color vision deficiencies. Several browser extensions and online tools exist for this purpose, and Elementor also features a built-in color blindness simulator.
Empathy plays a key role in accessible design. Putting yourself in the shoes of users with differing abilities fosters a deeper understanding of their needs.
Resources and Tools
Let’s look at some helpful resources to ensure your color choices are accessible:
- Contrast Checkers: Several online tools (like WebAIM’s Color Contrast Checker: https://webaim.org/resources/contrastchecker/) allow you to input your text and background colors and will indicate whether they meet WCAG contrast requirements.
- Accessible Color Palette Generators: Websites like Who Can Use: https://venngage.com/tools/accessible-color-palette-generator help you build color palettes that prioritize accessibility from the start.
Best Practices for Text Color Choices
Readability is Key
Your text color choices should never sacrifice readability. While a vibrant color scheme might be appealing, it’s only useful if your users can read the content. Here’s what to keep in mind:
- Background Matters: Text color needs to stand out clearly against the background color. Always use a contrast checker to verify this.
- Strong Contrast: Aim for high-contrast combinations, especially for essential text like body copy and navigation labels.
Branding and Color Psychology
Colors have the power to evoke emotions and strongly influence how your brand is perceived. Let’s consider how to align your text colors with your website’s purpose:
- Brand Palette: Incorporate your existing brand colors into your text styling to promote brand recognition and a cohesive look.
- Emotion: Understand the psychology of color. Warm colors (reds, oranges, yellows) convey energy and excitement, while cooler colors (blues, greens, purples) tend to be more calming.
Hierarchy and Emphasis
Use color to create a clear visual hierarchy on your pages. This helps guide the user’s eye and signals the importance of different text elements:
- Headings: Headings often work well with bolder or darker colors to stand out from the body text.
- Calls to Action: Make buttons and other important links pop with contrasting colors that attract attention.
- Subtlety: Use less saturated colors or smaller font sizes for less important text elements
Don’t Overdo It
A little color goes a long way! Here’s why simplicity is often best:
- Overwhelming: Too many colors can make your website busy and difficult to read.
- Consistency: A limited, well-chosen color palette creates a more harmonious and polished look.
Elementor: Styling Text Intuitively
Introducing the Elementor Visual Editor
Elementor’s core strength lies in its drag-and-drop visual editor. This editor gives you real-time control over your website’s design, including text color, without writing a single line of CSS (unless you want to). This approach empowers those without coding experience to create beautiful, color-rich websites.
Text Color Controls in Elementor Widgets
Nearly all Elementor widgets that contain text (headings, paragraphs, buttons, etc.) feature intuitive color picker controls within their styling options. Let’s illustrate this:
- Select a Widget: Choose any text-based widget on your page.
- Styling Tab: Navigate to the widget’s “Style” tab in the Elementor editing panel.
- Color Picker: Locate the “Text Color” option and click on it to reveal a user-friendly color picker.
- Choose Freely: You can select from a color palette, enter hex codes, or use the eyedropper tool to match existing colors on your website perfectly.
This streamlined workflow drastically speeds up your text color experimentation and implementation.
Global Style Settings
Elementor offers global style settings that let you define default colors for headings, body text, links, and more. This has several benefits:
- Consistency: Maintain a cohesive look across your entire website with ease.
- Time-Saver: Change site-wide colors with a few clicks instead of editing individual widgets.
Custom CSS Capabilities
While Elementor’s visual tools are incredibly powerful, you may want to fine-tune them with custom CSS. Elementor allows you to add custom CSS code directly to widgets, pages, or site-wide, giving you that extra level of control when needed.
Bonus: Advanced Techniques & Tools
Color Gradients
CSS gradients let you create smooth transitions between multiple colors within your text. This can produce eye-catching and memorable effects:
CSS
h1 {
background: linear-gradient(to right, #FF0000, #FFA500, #FFFF00);
-webkit-background-clip: text;
-webkit-text-fill-color: transparent;
}
To ensure color gradients display within your text, as in the example above, you’ll often need specific -webkit- prefixes for compatibility across different browsers.
Gradients offer a unique opportunity to add depth and visual interest to headings or call-to-action elements.
Text Shadows and Text Strokes
Let’s add some dimension to your text:
Text Shadows (text-shadow) Create subtle shadow effects behind your text, improving readability against certain backgrounds or adding a touch of style.
CSS
p {
text-shadow: 2px 2px 5px rgba(0, 0, 0, 0.5);
}
Text Strokes (-webkit-text-stroke) Add outlines to your text for increased emphasis or a bolder aesthetic.
CSS
h2 {
-webkit-text-stroke: 1px black;
color: white;
}
Use text shadows and strokes judiciously. Subtlety is often best, but bold effects can shine in specific use cases like large, decorative titles.
Color Functions (if space allows)
CSS provides functions like lighten(), darken(), saturate(), and others to manipulate colors on the fly. These are especially handy within preprocessors like Sass to dynamically calculate color variations based on your color theme.
CSS
/* Example using the lighten() function */
button:hover {
background-color: lighten(#006400, 10%); /* Lightens the green by 10% on hover */
}
140 Color Names and hex value Supported by All Browsers:
Conclusion
Throughout this guide, we’ve explored the world of text color in CSS. You’ve learned the fundamentals of the color property, different ways to express color values and methods for targeting specific elements within your website. We touched on accessibility, best practices, and even a sprinkle of advanced techniques.
Remember, color is a potent tool in your web design arsenal. Use it strategically to:
- Enhance readability
- Guide the user’s eye
- Evoke emotions and align with your brand
- Create a visually captivating and memorable website
If you’re looking to streamline the process of adding and styling text color, consider a powerful website builder like Elementor. Its intuitive visual interface and integration with optimized hosting can empower you to create beautiful, color-rich websites without needing extensive coding knowledge.
Looking for fresh content?
By entering your email, you agree to receive Elementor emails, including marketing emails,
and agree to our Terms & Conditions and Privacy Policy.