Table of Contents
Why is this important? Imagine a website designed for a desktop screen. When viewed on a mobile phone, everything might appear too small, making text difficult to read and buttons hard to tap. Using ‘vh’ helps address this problem. By setting a hero section to 100vh, for example, you ensure it will always fill the user’s screen perfectly, delivering an optimal viewing experience regardless of the device.
Let’s dive deeper into the ‘vh’ unit and uncover its full potential for responsive web design!
Understanding the Viewport
Before we master the ‘vh’ unit, we need a solid grasp of the browser viewport. Think of the viewport as the visible area of your website within the browser window. It’s important to note that the viewport is sometimes the same size as your device’s physical screen. Factors like browser toolbars, scrollbars, and whether the browser is maximized or resized can all influence viewport dimensions.
Why does this matter? Picture opening a website on your desktop and then on your phone. The same website needs to fit within vastly different viewport sizes. Understanding how the viewport works is the first step in using ‘vh’ to build websites that automatically adjust to these variations.
Grow Your Sales
- Incredibly Fast Store
- Sales Optimization
- Enterprise-Grade Security
- 24/7 Expert Service
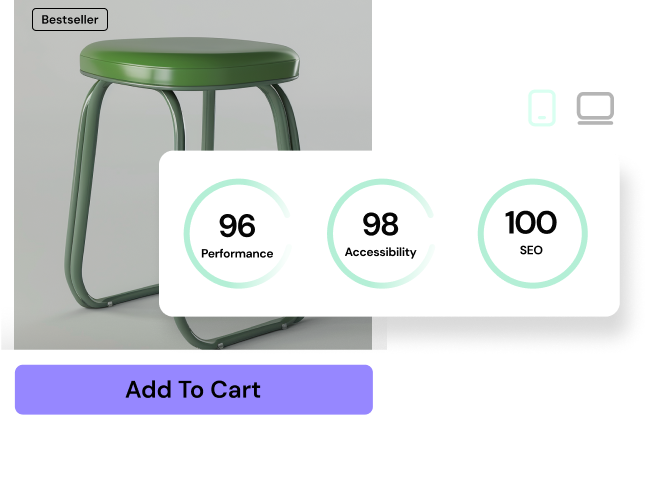
- Incredibly Fast Store
- Sales Optimization
- Enterprise-Grade Security
- 24/7 Expert Service
- Prompt your Code & Add Custom Code, HTML, or CSS with ease
- Generate or edit with AI for Tailored Images
- Use Copilot for predictive stylized container layouts
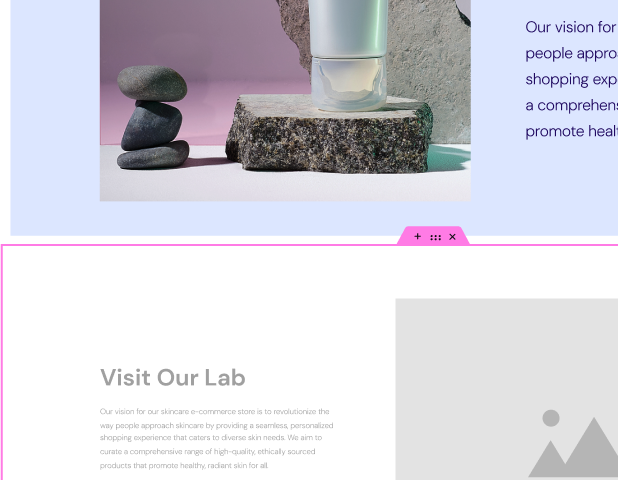
- Prompt your Code & Add Custom Code, HTML, or CSS with ease
- Generate or edit with AI for Tailored Images
- Use Copilot for predictive stylized container layouts
- Craft or Translate Content at Lightning Speed
Top-Performing Website
- Super-Fast Websites
- Enterprise-Grade Security
- Any Site, Every Business
- 24/7 Expert Service
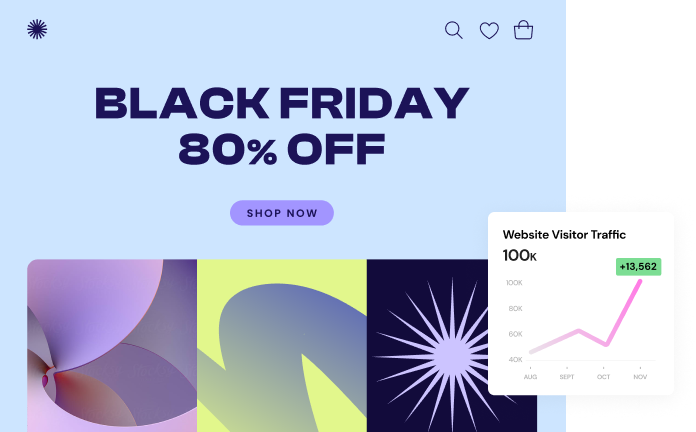
Top-Performing Website
- Super-Fast Websites
- Enterprise-Grade Security
- Any Site, Every Business
- 24/7 Expert Service
- Drag & Drop Website Builder, No Code Required
- Over 100 Widgets, for Every Purpose
- Professional Design Features for Pixel Perfect Design
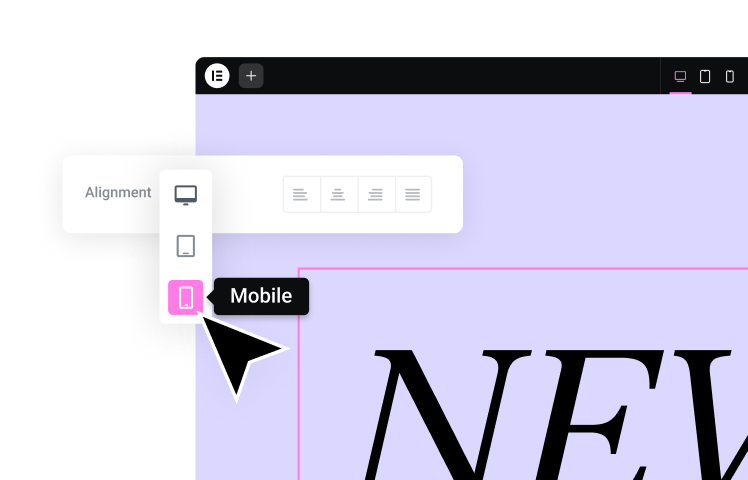
- Drag & Drop Website Builder, No Code Required
- Over 100 Widgets, for Every Purpose
- Professional Design Features for Pixel Perfect Design
- Marketing & eCommerce Features to Increase Conversion
- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking
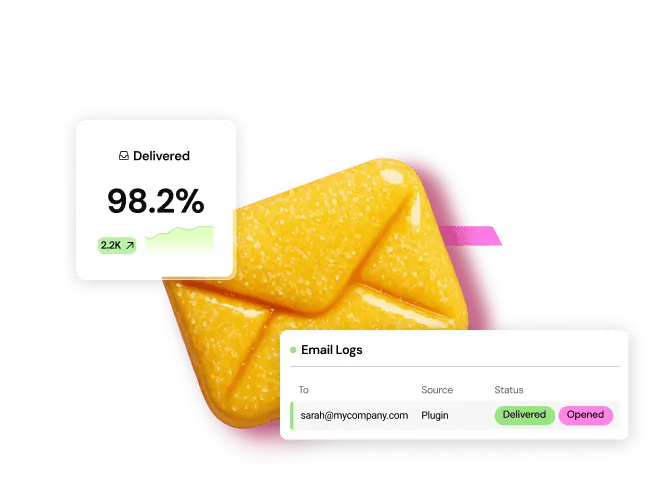
- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking
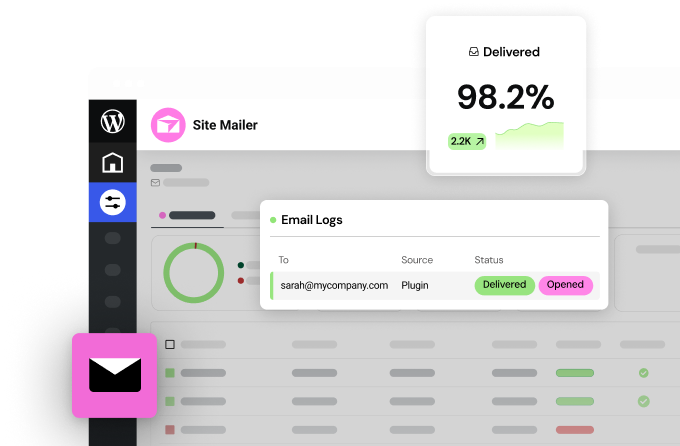
- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking
Core Concepts of vh
The magic of ‘vh’ lies in its simplicity. 1vh represents 1% of the viewport’s height. So, if a user’s browser window is 800 pixels tall, an element set to a height of 50vh will be 400 pixels tall (50% of 800). If they resize their browser window, the element’s height will dynamically adjust along with the changing viewport.
Let’s illustrate this with a simple code example:
HTML
<div class="hero-section">
<h1>Welcome to My Website</h1>
</div>
CSS
.hero-section {
height: 100vh;
background-color: #f0f0f0;
}
In this example, the hero section will always occupy the full height of the viewport, no matter what device the user is using.
Key Points
Relative Sizing: ‘vh’ is a relative unit of measurement, meaning its size is calculated in relation to the viewport height. This is in contrast to absolute units like pixels (px), which have a fixed size.
Other Viewport Units: While we’re focusing on ‘vh’ (viewport height), there are related units:
- vw: Viewport width (1vw = 1% of the viewport width)
- vmin: The smaller of viewport height or width
- vmax: The larger of viewport height or width
Advantages over Other Units
Using ‘vh’ for responsive design offers several advantages compared to alternatives like pixels, percentages, or ems:
- True Responsiveness: Elements sized with ‘vh’ will scale seamlessly across devices, ensuring your content is always displayed optimally.
- Easy Calculations: ‘vh’ makes it simple to create full-height sections or elements that take up a specific percentage of the screen.
Practical Applications of vh
Full-Screen Elements
One of the most common uses of ‘vh’ is creating elements that span the full viewport height. Let’s look at some prime examples:
- Hero Sections: A hero section is typically the first thing a visitor sees on your website. Using height: 100vh ensures your hero—with its eye-catching image, powerful headline, and call to action—makes a bold impact on any screen size.
- landing pages: Often designed to be visually striking and persuasive, landing pages can greatly benefit from ‘vh. Setting sections to precise viewport percentages allows you to control the flow of information and guide the user’s eye.
- Background Images: Want a background image to cover the screen? ‘vh perfectly’ comes to the rescue! Combine it with properties like background-size: cover to achieve this effect.
Vertically Centered Content
Vertically centering elements used to be a web design headache, often involving complex CSS workarounds. With ‘vh’, it becomes remarkably straightforward. Here’s a basic example:
HTML
<div class="centered-content">
<p>This content is perfectly centered!</p>
</div>
CSS
.centered-content {
height: 50vh;
display: flex;
align-items: center;
justify-content: center;
}
In this example, we set a container to 50vh and use flexbox properties for effortless vertical centering.
Sticky Elements
Want a navigation menu or footer to stay “stuck” to the viewport even when the user scrolls? ‘vh’ can help:
- Sticky Footers: A sticky footer that always remains at the bottom of the page (when content is short) is achievable with ‘vh’, min-height, and flexbox.
- Navigation Menus: You can create sticky navigation that stays at the top of the screen, ensuring important links are always accessible.
- Dynamic Scaling: ‘vh’ isn’t just about full-screen elements. You can use it to scale elements proportionally to the viewport. For instance, typography (font sizes) or images to subtly adjust based on screen size, providing a more comfortable reading experience across devices.
- Parallax Effects: Parallax effects, where different elements scroll at varying speeds, create an illusion of depth and can add a touch of visual interest to your website. ‘vh’ can be used to define how these elements react to scrolling based on the viewport’s dimensions.
- Modal Overlays: Modals (or popups) often need to take over the screen and sometimes be vertically centered. ‘vh’, combined with techniques for centering and creating full-screen overlays, is perfect for this task.
Important Note: While ‘vh’ is incredibly useful, there are cases where other CSS properties might be more suitable. For example, if content naturally defines its own height (like a paragraph of text), there’s usually no need to force it using ‘vh’.
VH in Combination with Other CSS Properties
Min-height and max-height
While ‘vh’ sets a relative height, sometimes you might need more control.
- min-height: Sets a minimum height for an element, preventing it from shrinking below a certain size even if the viewport is very small.
- max-height: Sets a maximum height, ensuring an element won’t grow too large on wide screens.
Example: You want a banner always to take up at least 50% of the screen height but no more than 600 pixels tall:
CSS
.banner {
height: 50vh;
min-height: 300px;
max-height: 600px;
}
calc()
The CSS calc() function lets you perform calculations, making ‘vh’ even more versatile. Imagine creating a sidebar that takes up 80% of viewport height, minus a 50px top margin:
CSS
.sidebar {
height: calc(80vh - 50px);
}
Margin and Padding with ‘vh’
You can use ‘vh’ for margin and padding as well. This might come in handy for creating spacing that scales proportionally to the screen size. For instance:
CSS
.section-title {
margin-bottom: 2vh; /* Creates a consistent margin on different screens */
}
Important Considerations:
- Overflow: If the content within a ‘vh’-sized element exceeds the available space, you might encounter overflow issues. Use properties like overflow: hidden or overflow: scroll to manage this behavior.
- Mobile Address Bars: On some mobile browsers, the address bar can shrink and expand as the user scrolls, dynamically changing the viewport height. This can sometimes lead to unexpected layout shifts. Be aware of this potential issue during testing.
Responsive Design with vh
In today’s world, where websites are accessed on a dizzying array of devices with varying screen sizes, responsive design isn’t just a “nice to have” – it’s essential. ‘vh’ is a powerful tool to help you achieve this goal. Let’s explore how:
Media Queries and Viewport Breakpoints
Media queries allow you to apply different CSS rules based on viewport dimensions. Common breakpoints target ranges like mobile, tablet, and desktop screen sizes. Here’s how ‘vh’ can be used within media queries:
CSS
@media (max-width: 768px) {
.hero-section {
height: 60vh; /* Adjust hero height for smaller screens */
}
}
Mobile-First vs. Desktop-First Approach
- Mobile-First: Start with base styles for smaller screens, then use media queries to layer on styles for larger viewports. This approach can help prioritize content and optimize for the growing number of mobile users.
- Desktop-First: Begin with styles for desktops, then use media queries to adjust elements for smaller viewports.
Both approaches are valid, and your choice often depends on your website’s target audience and content structure.
Optimizing for Various Devices
Consider how your ‘vh’ usage impacts the user experience on different devices:
- Mobile: Be mindful of potential address bar resizing issues and ensure touch targets (buttons, links) are sized appropriately for easy interaction.
- Tablet: Aim for a balance of visual impact and readability with ‘vh’-based elements.
- Desktop: You have more flexibility with larger screens, but avoid making elements too overwhelming in their scale.
Elementor responsive editing tools
While ‘vh’ gives you a lot of power, using it effectively within complex layouts can be challenging. This is where Elementor shines. Elementor’s visual, drag-and-drop interface and responsive editing tools make tweaking your designs for different breakpoints incredibly intuitive. You can directly modify how elements using ‘vh’ will appear on mobile, tablet, and desktop – no code editing required (although you can add custom CSS if needed)!
vh for Advanced Web Design
Complex Layout Calculations
When you need precise layout control, ‘vh’ in tandem with the calc() function becomes your best friend. Say you want a layout with a fixed header, a content area taking up 80% of the remaining screen space, and a sticky footer:
CSS
.header {
height: 100px;
}
.content-area {
height: calc(80vh - 100px); /* Subtract header height */
}
.footer {
height: 50px;
}
Creative User Interface Elements
vh’ can power innovative UI elements that react to the viewport size. Imagine a navigation sidebar that smoothly slides in when triggered, taking up a specific percentage of the screen height. You may want to create interactive elements that change size or position based on how far the user has scrolled.
Animations and Transitions Powered by ‘vh’
Add another dimension of visual interest by combining ‘vh’ with CSS animations and transitions. Here are some ideas:
- Elements that scale in or out of view as the user scrolls.
- Background elements subtly shift position, creating a sense of movement and depth.
- Progress indicators or loading bars that dynamically adjust their length based on the viewport height.
Important Reminders:
- Use with Intention: Don’t overcomplicate your designs with excessive ‘vh’-driven elements. Apply these advanced techniques thoughtfully, focusing on enhancing the user experience, not detracting from it.
- Accessibility: Always consider accessibility when using dynamic layout techniques. Provide keyboard navigation options, use appropriate ARIA attributes, and test to ensure your website functions well for users with assistive technologies.
- Performance: Be mindful of the potential performance impact of complex ‘vh’-based calculations and animations, especially on lower-powered devices. Optimize as needed.
Browser Compatibility and Troubleshooting
Unfortunately, older browsers (particularly some versions of Internet Explorer and mobile browsers) have had inconsistent or buggy implementations of the ‘vh’ unit. While browser support has vastly improved in recent years, it’s still wise to be aware of potential problem areas and mitigation strategies.
Common Issues
- Incorrect height calculations: In some browsers, ‘vh’ might not calculate correctly if the page hasn’t been fully loaded or is within specific contexts (like within iframes).
- Address bar woes: The resizing mobile browser address bar can cause ‘vh’ elements to jump or jitter unexpectedly.
- 100vh overflow on iOS: There was a long-standing bug in iOS Safari where setting an element to 100vh could cause its content to overflow.
Workarounds and Troubleshooting
- Polyfills: JavaScript polyfills exist to mimic ‘vh’ behavior in older browsers that lack proper support. However, modern browsers generally won’t need these.
- JavaScript Fallbacks: In specific cases, you might consider JavaScript solutions that calculate viewport height and apply it dynamically to elements.
- Alternative CSS Units: If complex layouts are proving difficult with ‘vh’ due to browser issues, sometimes a combination of percentages, fixed heights (px), and media queries can be a more compatible solution.
- Thorough Testing: Test your website on a range of devices and browsers (especially older versions), and visually inspect how your ‘vh’-based elements behave.
The good news: As browser vendors prioritize standards compliance, these compatibility issues are becoming less frequent. Still, it’s good practice to stay aware of them and test accordingly!
Important Note: When making troubleshooting decisions, consider your target audience and the tradeoffs between cutting-edge design and ensuring a smooth experience for users on older browsers.
Elementor Website Builder vs. Coding from Scratch
- Speed of Development: Elementor significantly accelerates the website-building process. Creating a custom-coded website from the ground up takes considerably more time and expertise.
- Ease of Use: Elementor’s visual interface and intuitive workflow make it accessible to non-technical users. Coding a website requires proficiency in HTML, CSS, and potentially JavaScript.
- Design Control: While Elementor offers a high degree of design freedom, fully custom-coded websites ultimately provide the most flexibility if you have the development resources.
The Future of VH and Web Design
Evolution of Viewport Units
While ‘vh’ is incredibly useful, there’s room for additional viewport-based units. Imagine a ‘vmin’ equivalent for width (vwmin) or units based on the viewport’s aspect ratio. These could open up even more possibilities for dynamic, responsive layouts.
Emerging Design Trends
Web design trends are constantly shifting. ‘vh’ will likely play a significant role in trends such as:
- Neumorphism and Soft UI: ‘vh’ can be used to create subtle depth effects and element scaling that align with this aesthetic.
- Scrolling Effects: Expect to see more creative uses of ‘vh’ for scroll-triggered animations, parallax effects, and interactive storytelling experiences on websites.
- Variable fonts: Combining ‘vh’ with variable fonts, which can fluidly change size and weight, could lead to highly adaptable typography that perfectly scales with the viewport.
Accessibility Considerations
As designers focus on creating inclusive web experiences, the way ‘vh’ is used will need to evolve in tandem with accessibility best practices. This includes ensuring dynamic scaling works well for users with visual impairments and providing alternative experiences when necessary.
The future looks bright for ‘vh’! Its versatility and ability to power responsive designs guarantee it’ll remain an essential tool in the web developer’s toolkit.
Conclusion
Throughout this guide, we’ve uncovered the power of the ‘vh’ CSS unit in creating responsive and flexible web designs. From full-screen hero sections to dynamic scaling and interactive elements, ‘vh’ opens up a world of possibilities when building websites that adapt seamlessly to different devices.
Remember, the true effectiveness of ‘vh’ is amplified when paired with the right tools and infrastructure:
Intuitive Website Builder: Elementor’s visual editor and responsive controls streamline the process of implementing your ‘vh’ based designs and previewing them across various screen sizes.
By understanding the ‘vh’ unit, employing responsive design principles, and leveraging solutions like Elementor, you’re well-equipped to build stunning, user-friendly websites that shine on any device.
Originally posted 2020-03-27 20:02:00.
Looking for fresh content?
By entering your email, you agree to receive Elementor emails, including marketing emails,
and agree to our Terms & Conditions and Privacy Policy.