Table of Contents
This guide will unlock the secrets to becoming a web developer. We’ll cover everything from the essential building blocks of websites to crafting stunning designs that engage users. You’ll learn about different development paths, powerful tools of the trade, and how to land your dream job in this dynamic field.
But why learn web development? Here are a few compelling reasons:
- High Demand: Companies across the globe need skilled web developers to build and maintain their online presence.
- Flexibility and Creativity: Web development blends technical skills with design and problem-solving flair, offering a fulfilling mix of logic and artistry.
- Diverse Opportunities: Specialize in front-end (what users see) and back-end (powering functionality), or become a full-stack developer handling it all.
- Accessibility: Numerous online resources, coding boot camps, and self-study options make learning web development achievable for everyone.
Mastering the Core Fundamentals
HTML: The Structural Backbone
Think of a website as a house. HTML (Hypertext Markup Language) is its foundation, walls, and roof. It’s the code that defines the basic content and structure of a web page. Here’s what you need to know:
- Elements and Tags: HTML uses elements enclosed in tags to tell the browser what to display. For example: <h1>This is a Heading</h1> or <p>This is a paragraph of text.</p>
- Semantic HTML Using the correct HTML elements makes your website more accessible and search engine friendly. Choose tags like <header>, <nav>, <main>, <article>, and <footer> to give your code meaning and context.
- Attributes: Attributes add extra information to elements; for example, a <img> tag uses the src attribute to specify the image location and the alt attribute to provide a text description for accessibility.
- Nesting Elements: Elements can be placed inside other elements to create hierarchy and structure. A <div> might contain a <h1> heading followed by several <p> paragraphs.
Grow Your Sales
- Incredibly Fast Store
- Sales Optimization
- Enterprise-Grade Security
- 24/7 Expert Service
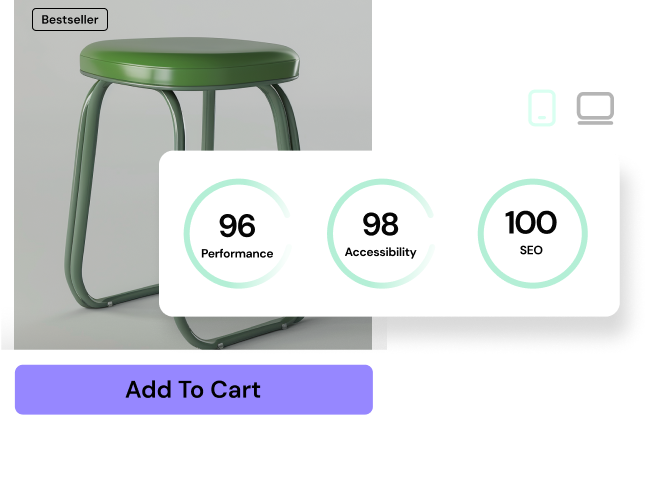
- Incredibly Fast Store
- Sales Optimization
- Enterprise-Grade Security
- 24/7 Expert Service
- Prompt your Code & Add Custom Code, HTML, or CSS with ease
- Generate or edit with AI for Tailored Images
- Use Copilot for predictive stylized container layouts
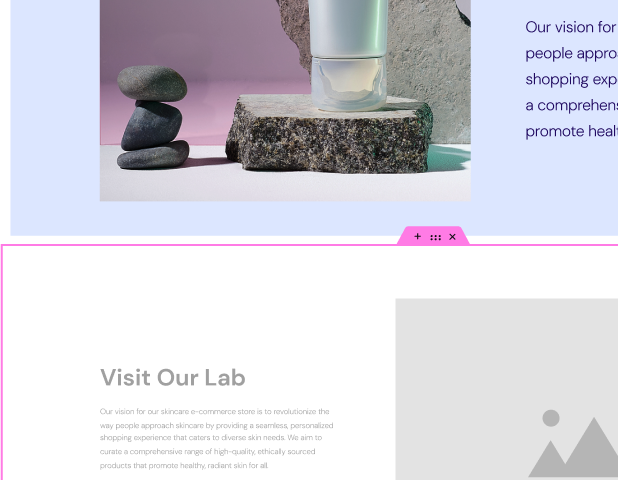
- Prompt your Code & Add Custom Code, HTML, or CSS with ease
- Generate or edit with AI for Tailored Images
- Use Copilot for predictive stylized container layouts
- Craft or Translate Content at Lightning Speed
Top-Performing Website
- Super-Fast Websites
- Enterprise-Grade Security
- Any Site, Every Business
- 24/7 Expert Service
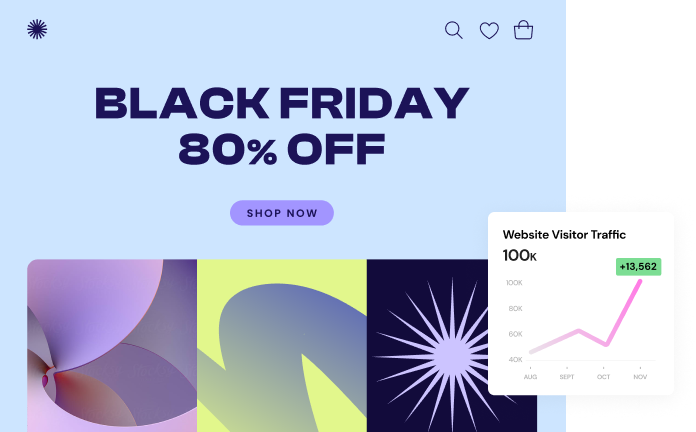
Top-Performing Website
- Super-Fast Websites
- Enterprise-Grade Security
- Any Site, Every Business
- 24/7 Expert Service
- Drag & Drop Website Builder, No Code Required
- Over 100 Widgets, for Every Purpose
- Professional Design Features for Pixel Perfect Design
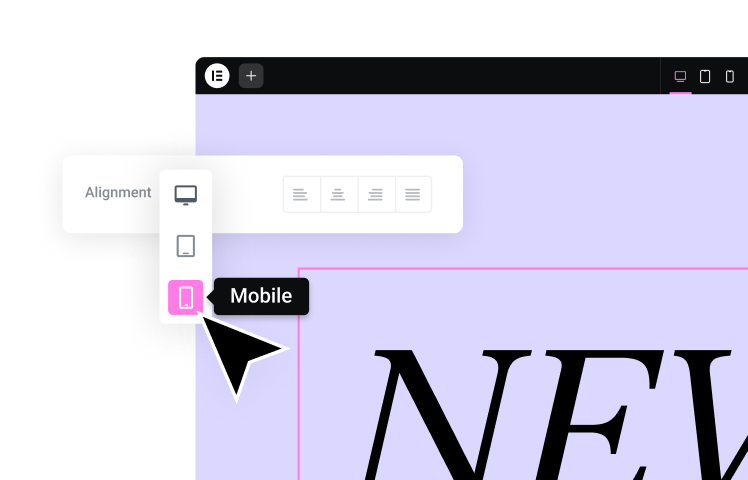
- Drag & Drop Website Builder, No Code Required
- Over 100 Widgets, for Every Purpose
- Professional Design Features for Pixel Perfect Design
- Marketing & eCommerce Features to Increase Conversion
- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking
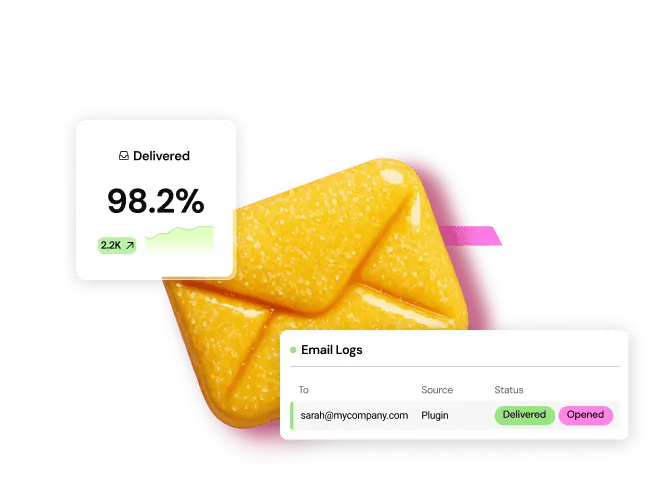
- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking
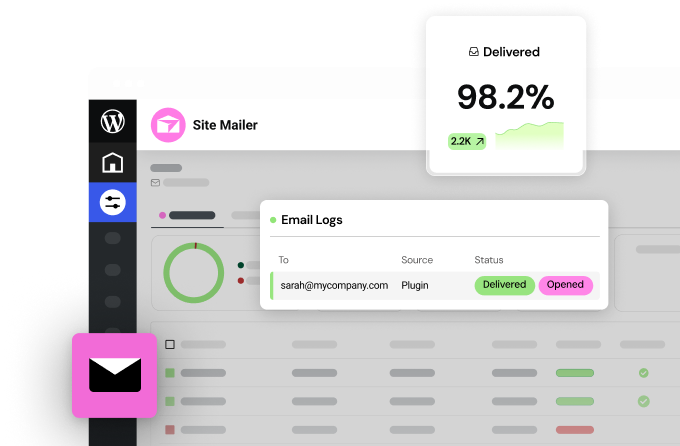
- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking
CSS: Styling and Presentation
If HTML is the skeleton of a website, CSS (Cascading Style Sheets) is the skin, clothing, and makeup. It controls the layout, colors, fonts, and overall look and feel. Let’s break down the key concepts:
- Selectors: CSS targets specific HTML elements to apply styles. You can use element selectors (targeting all paragraph elements, for example), class selectors (for groups of elements), and ID selectors (for unique elements).
Properties and Values
CSS has numerous properties to style elements. Common ones include:
- color: Changes text color
- background-color: Sets background color
- font-family: Specifies fonts
- margin: Creates space around elements
- padding: Creates space within an element
The Box Model: Every element in CSS is treated as a box. Understanding the box model (content, padding, border, margin) is crucial for precise layouts.
Responsive Design: Modern websites need to look great on all devices. CSS media queries let you adjust styles based on screen size, ensuring your website adapts seamlessly across desktops, tablets, and smartphones.
JavaScript: Bringing Interactivity
JavaScript is the programming language that makes websites truly come alive. It enables interactive elements, animations, and the ability to respond to user input. Here’s what you should focus on initially:
- Variables and Data Types: JavaScript stores data using variables. Understanding data types like strings (text), numbers, booleans (true/false), and arrays (lists of data) is essential.
- Functions are reusable blocks of code that perform specific tasks. They make your code more organized and efficient.
- The DOM: JavaScript sees a web page using the Document Object Model (DOM). You’ll learn to use JavaScript to manipulate elements, add content, change styles, and react to events (like button clicks).
- Events: Events are what trigger things to happen on a web page. Learn how to handle events like clicks, mouse hovers, form submissions, and more.
Choosing Your Development Path
Web development offers exciting specializations depending on where your interests and talents lie. Let’s explore the major paths:
Front-End Development
Front-end developers are the masters of the user experience (UX). They focus on the visual aspects of websites and how people interact with them. Here’s a deeper look:
- Popular Frameworks To streamline front-end development, frameworks like React, Angular, and Vue.js provide structure, pre-built components, and efficient ways to manage complex interfaces. Understanding the core concepts behind one of these frameworks will greatly enhance your skillset.
- UI Libraries and Components: Libraries like Bootstrap and Tailwind CSS offer pre-designed elements (buttons, navigation menus, etc.), speeding up development and ensuring visual consistency.
- Design Sensibility While not all front-end developers are designers, having an eye for aesthetics, intuitive layouts, and a strong understanding of user experience principles will make you stand out.
Back-End Development
Back-end developers deal with the “behind-the-scenes” functionality of a website. They focus on the server side, databases, and logic that powers everything the user doesn’t directly see. Let’s break it down:
- Server-Side Languages: Powerhouses like Python, Java, PHP, Ruby on Rails, and Node.js write the logic behind web applications, process data, and communicate with databases.
- Databases: Understanding how to store, retrieve, and manage data with SQL (relational databases) or NoSQL (non-relational databases) is crucial for any back-end developer.
- APIs: APIs (Application Programming Interfaces) allow different parts of a website, or even separate applications, to communicate. Back-end developers often build APIs to expose data or functionality.
- Full-Stack Development
Full-stack developers have a deep understanding of both front-end and back-end technologies. While specializing has its merits, being a full-stack developer offers versatility and a holistic view of how websites work. It can be a satisfying path for those who want to see their projects through from beginning to end.
Building Your Toolkit
Code Editors and IDEs
Think of code editors as your workshop. They are specialized text editors with features that make writing and debugging code much easier. Let’s look at some options:
- Code Editors: Popular choices like Visual Studio Code (VS Code), Sublime Text, and Atom offer syntax highlighting (different colors for code elements), code completion (suggesting code as you type), and helpful extensions that add even more functionality.
- IDEs: Integrated Development Environments (IDEs) take things a step further. They often include advanced features like built-in debugging tools, version control integration, and the ability to run your code directly within the environment. WebStorm and PHPStorm are powerful IDEs popular with web developers.
Version Control (Git)
Think of version control as a time machine for your code. Git is the most popular version control system, enabling you to:
- Track Changes: Git records every modification, allowing you to revisit older versions of your code if needed.
- Experiment with Confidence: Create “branches” to try out new features without disrupting your main codebase.
- Collaboration: Git makes it easy for multiple developers to work on the same project simultaneously, streamlining teamwork.
- Understanding the Basics: Focus on learning key Git commands like add, commit, push, pull, and how to create and merge branches.
Debugging Tools
No matter how skilled you become, encountering bugs (errors in your code) is inevitable. Thankfully, powerful tools help you find and fix problems:
Browser Developer Tools
Built into all modern web browsers (Chrome, Firefox, Edge, etc.), developer tools offer a treasure trove of features:
- Console: Displays errors, warnings, and logs that you can use to pinpoint issues.
- Inspector: Lets you examine and modify your HTML and CSS live, helping you identify layout or styling problems.
- Network Tab: Monitors web requests and responses, useful for debugging data fetching or API issues.
- Debugging Extensions: Many code editors and IDEs have built-in debuggers or extensions that provide advanced debugging features like setting breakpoints (pausing code execution), stepping through code line by line, and inspecting variable values.
Debugging Techniques:
- Rubber Duck Debugging: Talking through your issue aloud, even if it’s to an inanimate object, can force you to clarify your thinking and often pinpoint the problem.
- Strategic Logging: Using console.log statements (or equivalent in server-side languages) to print variable values at different points in your code helps track execution flow.
- Start Simple, Then Isolate: Begin by checking for typos and common syntax errors. Next, try to isolate the problem to a specific function or block of code.
Web Developer Salary: A Comprehensive Guide
Web developers play a pivotal role in building and maintaining the digital landscape. With the ever-growing reliance on websites and applications, the demand for skilled web developers remains consistently high, leading to attractive salary packages. This article analyzes the factors affecting a web developer’s salary and provides insights into potential earnings across different locations, experience levels, and specializations.
Factors Influencing Web Developer Salaries
- Location: Geographical location plays a significant role in determining salary potential. Major tech hubs like Silicon Valley, New York City, and Tel Aviv tend to offer higher salaries due to increased competition and higher living costs.
- Experience: As with most professions, experience directly correlates to higher earning potential. Developers with several years of experience command higher salaries than those just starting.
- Specialization: Web development encompasses various specializations – frontend, backend, full-stack, mobile development, and more. Expertise in a high-demand niche can lead to a premium in compensation.
- Industry: The industry in which a web developer works can affect salary. Tech companies generally pay more than traditional sectors like healthcare or education.
- Company Size and Type: Larger, well-established companies might offer better salary packages and benefits compared to smaller firms or startups.
- Education and Skills: Formal education and certifications can be influential, but proficiency in relevant programming languages and frameworks is paramount.
- Negotiation Skills: Effectively negotiating your salary based on your experience, skills, and market value can significantly impact your final compensation.
Salary Ranges
While specific figures will vary, here’s a broad look at web developer salary ranges based on experience:
- Entry-Level (0-2 years experience): $50,000 – $85,000 annually
- Mid-Level (3-5 years experience): $80,000 – $120,000 annually
- Senior-Level (5+ years experience): $110,000 – $160,000+ annually
Increasing Your Earning Potential
- Upskilling: Stay up-to-date with the latest technologies and frameworks. Expand your knowledge base to become more valuable in the job market.
- Build a Strong Portfolio: Showcase your best projects to potential employers, highlighting your problem-solving and development skills.
- Network: Connect with other web developers, attend industry events, and cultivate relationships that lead to higher-paying job opportunities.
- Consider Freelance Work: Supplement your income with freelance projects or explore the flexible lifestyle it offers.
Web development offers a financially rewarding career path. Remember, salary figures are fluid and influenced by the factors outlined above. By investing in continuous skill development, proactively seeking opportunities, and strategically positioning yourself in the market, you can achieve a successful and well-compensated career in web development.
Leveling Up with Design and User Experience
Design Principles for the Web
While traditional graphic design principles apply, the web brings its own unique considerations. Let’s focus on a few key aspects:
- Color Theory: Understanding how colors evoke emotions and create contrast is vital for a visually appealing website. Explore color palettes and online tools for generating harmonious color schemes.
- Typography: Choosing the right fonts greatly impacts readability and the overall feel of your website. Font size, hierarchy (using headings like H1 and H2), and line spacing all play a role.
- Layout and Hierarchy: Well-structured layouts guide the user’s eye. Think about the grid, negative space (whitespace), and visual weight to create a clear information flow.
- Mobile-First Design: With most web traffic coming from mobile devices, designing for smaller screens first and then adapting to larger ones ensures an optimal experience for everyone.
UX Best Practices
User experience (UX) focuses on how people interact with your website and how it makes them feel. Let’s break down some essential practices:
- User Research: Understanding your target audience through methods like surveys, interviews, or usability testing is crucial for creating user-centered websites.
- Journey Mapping: Visualizing a user’s steps as they interact with your website helps pinpoint bottlenecks or pain points in the experience.
- Navigation: Your site’s structure and menus should be intuitive and easy to follow, allowing users to find what they need effortlessly.
- Clear Calls to Action: Buttons and links should be prominently placed and contain compelling text guiding users to take desired actions (signing up, buying a product, etc.).
Building Your Portfolio and Landing Your First Job
Projects that Showcase Your Skills
Your portfolio is your most important asset when seeking work. It’s where you demonstrate not only what you can create but also how you approach problem-solving. Here’s what to include:
- Personal Projects: If you’re just starting, create passion projects that pique your interest. Experiment with different technologies and showcase your initiative.
- Freelance Work: Even small freelance projects offer real-world experience and add credibility to your portfolio.
- Open-Source Contributions: Participating in open-source projects demonstrates collaboration skills and allows potential employers to review your code directly.
Crafting a Compelling Portfolio Website
Your portfolio website should be a testament to your web development skills. Here’s what matters:
- Clean Design: Opt for a simple and professional design that highlights your work.
- Clear Project Descriptions: Go beyond visual screenshots. Explain your thought process, technologies used, and challenges faced.
- Easy Navigation: Make it easy for visitors to find what they’re looking for.
- Responsive: Ensure your portfolio website renders beautifully across all devices.
- Consider Using Elementor: If you want a visually appealing portfolio website, Elementor website builder is a fantastic option. It offers extensive design flexibility and showcases your understanding of this popular tool.
Networking within the Web Development Community
Building relationships is crucial for landing opportunities. Here are some ways to connect:
- Online Forums & Communities: Participate in discussions, ask questions, and help others. Many online platforms cater to web developers of all levels.
- Web Development Meetups: Attend local meetups to connect with other developers in your area.
- Code Repositories: Platforms like GitHub are not just for storing code but also opportunities to network with other developers.
Preparing for Technical Interviews
Technical interviews often assess your coding skills, problem-solving abilities, and understanding of web development concepts. Here’s how to prepare:
- Review Fundamentals: Brush up on your HTML, CSS, JavaScript, and relevant frameworks or languages.
- Practice Coding Challenges: Websites like LeetCode or HackerRank offer practice problems to sharpen your algorithmic thinking.
- Whiteboard Practice: Get comfortable solving coding problems on a whiteboard or in a text editor without running your code. Talk aloud through your thought process.
- Build Small Projects: Having code on hand to review and discuss demonstrates your ability to apply your skills to real-world problems.
- Review Common Questions: Research frequently asked interview questions and prepare your answers.
Freelance vs. Agency vs. In-House
Each work environment brings its own unique benefits and challenges. Let’s break down what to consider:
- Freelancing Offers flexibility, control over your projects, and the potential for higher earning potential. However, it requires strong self-discipline, client acquisition skills, and the ability to handle business aspects independently.
- Agency: This position provides exposure to diverse projects, a collaborative environment, and structured learning opportunities. Expect fast-paced work and the need to manage multiple clients.
- In-house: This option offers stability, focused work on a single product, and the chance to be part of a larger team. Potential downsides might include less varied work and slower technology adoption.
Continuous Learning and Growth
Staying Up-to-Date with Web Technologies
The web is a constantly evolving landscape. New technologies, frameworks, libraries, and best practices emerge all the time. Here’s why staying ahead of the curve is crucial:
- Marketability: Being proficient in in-demand technologies makes you more attractive to potential employers.
- Efficiency: New tools and frameworks often streamline development processes, saving you time and effort.
- Problem-Solving: Broad knowledge opens up new possibilities for solving complex development challenges.
- Security: Understanding emerging security threats helps you build more secure and resilient websites.
Online Resources, Courses, and Conferences
The internet is brimming with resources to aid your continuous learning. Let’s explore a few:
- Online Courses & Platforms: Platforms like Udemy, Coursera, Codecademy, Treehouse, and countless others offer structured courses on a wide range of web development topics.
- Web Development Blogs & Publications: Follow respected blogs and publications for industry news, tutorials, and in-depth analysis of new technologies.
- Documentation: The official documentation for languages, frameworks, and libraries is often the most reliable source for correct usage.
- Conferences: Attend web development conferences to learn from industry leaders, network, and explore the latest trends.
Conclusion
Web development is a journey, not a destination. By embracing the technologies, tools, and best practices outlined in this guide, you’ve taken significant strides toward becoming a successful web developer. Let’s recap some key takeaways:
- The Fundamentals are Key: A solid understanding of HTML, CSS, and JavaScript is the foundation upon which everything else is built.
- Choose Your Path: Explore front-end, back-end, or full-stack development to discover where your interests and talents lie.
- Tools Matter: Code editors, website builders like Elementor, and hosting solutions streamline your workflow and enhance your capabilities.
- User Experience is Paramount: Design websites that are not only beautiful but intuitive and enjoyable to use.
- Your Portfolio is Your Showcase: Invest time in creating a portfolio website that highlights your skills and unique projects.
- Network and Learn: Engage with web development communities, seek out mentors, and take advantage of the wealth of online learning resources.
Remember, the web development landscape is always changing. The most successful developers are those who possess an unwavering passion for learning and a drive to improve continuously. Embrace the challenges, stay curious, and never stop building!
Originally posted 2023-03-22 10:29:00.
Looking for fresh content?
By entering your email, you agree to receive Elementor emails, including marketing emails,
and agree to our Terms & Conditions and Privacy Policy.