Table of Contents
In this comprehensive guide, we’ll delve into the world of CSS background images, exploring everything from the basics to advanced techniques. Whether you’re a seasoned web developer or just starting, you’ll discover how to:
- Choose the right image formats
- Control image tiling and repetition
- Master precise positioning
- Create fixed and parallax effects
- Layer multiple images
- Use gradients for dynamic backgrounds
- Optimize images for lightning-fast loading times
If you’re ready to elevate your website designs, let’s dive in!
The Foundations of CSS Background Images
Let’s start by understanding the core concepts and properties that control how background images are displayed.
The background-image Property
The heart of CSS background image implementation is the background image property. This is where you tell the browser which image file to use as your background. Here’s the basic syntax:
CSS
selector {
background-image: url('path/to/your/image.jpg');
}
Selector
This is the HTML element where you want the background image to appear (e.g., body, a specific div, etc.).
url()
This function specifies the image’s location. The path can be:
- Relative: Relative to the location of your CSS file.
- Absolute: A full web address, including the protocol (http:// or https://).
Example:
CSS
body {
background-image: url('/images/background.png');
}
Important Note: Always enclose your image URL within single or double quotes to ensure proper browser interpretation.
File Formats
Web browsers support a range of image formats, but the most common ones for backgrounds are:
- JPEG (or JPG): is best for photographs or images with complex colors and gradients. It offers good compression.
- PNG: Great for images with transparency or when you need lossless compression (logos, graphics).
- GIF: Used for simple animations but generally less suitable for large background images.
- SVG: Scalable Vector Graphics are ideal for icons, illustrations, or patterns as they scale infinitely without loss of quality.
- WebP: is a modern format offering both lossy and lossless compression. It is often superior to JPEG and PNG in file size, but it has less universal browser support.
background-repeat
The background-repeat property dictates how a background image is tiled or repeated within its container element. Here are the possible values:
- repeat: The image repeats both horizontally and vertically (default behavior).
- repeat-x: The image repeats only horizontally.
- repeat-y: The image repeats only vertically.
- no-repeat: The image is displayed once without any repetition.
- space: The image is repeated, and any extra space is distributed evenly between the images.
- round: The image is repeated as much as needed to fill the space, but images are either squeezed or stretched to avoid partial tiles.
Example:
CSS
div {
background-image: url('pattern.png');
background-repeat: repeat-y;
}
Grow Your Sales
- Incredibly Fast Store
- Sales Optimization
- Enterprise-Grade Security
- 24/7 Expert Service
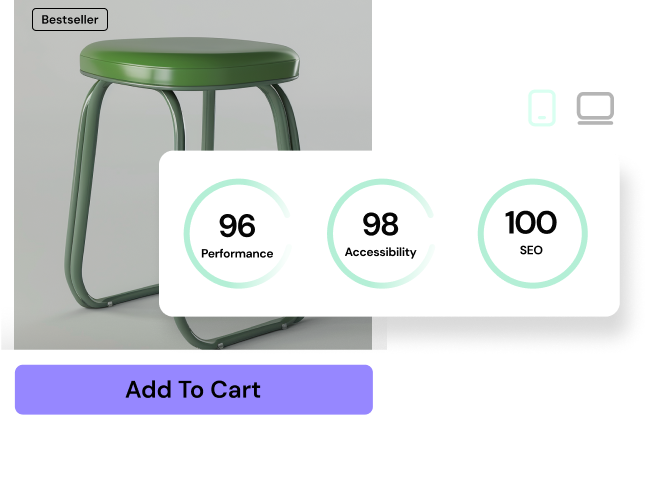
- Incredibly Fast Store
- Sales Optimization
- Enterprise-Grade Security
- 24/7 Expert Service
- Prompt your Code & Add Custom Code, HTML, or CSS with ease
- Generate or edit with AI for Tailored Images
- Use Copilot for predictive stylized container layouts
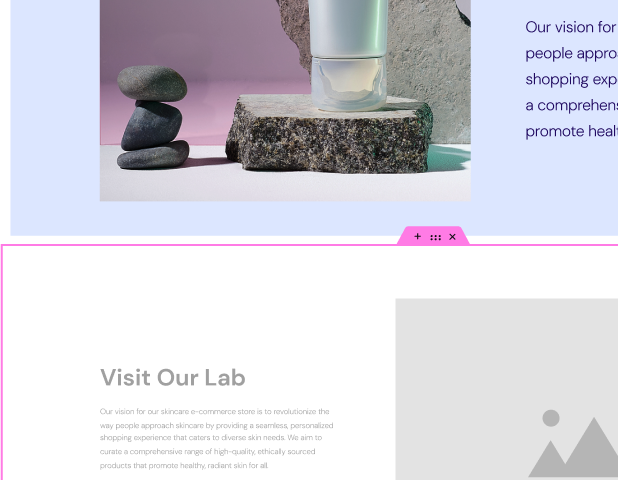
- Prompt your Code & Add Custom Code, HTML, or CSS with ease
- Generate or edit with AI for Tailored Images
- Use Copilot for predictive stylized container layouts
- Craft or Translate Content at Lightning Speed
Top-Performing Website
- Super-Fast Websites
- Enterprise-Grade Security
- Any Site, Every Business
- 24/7 Expert Service
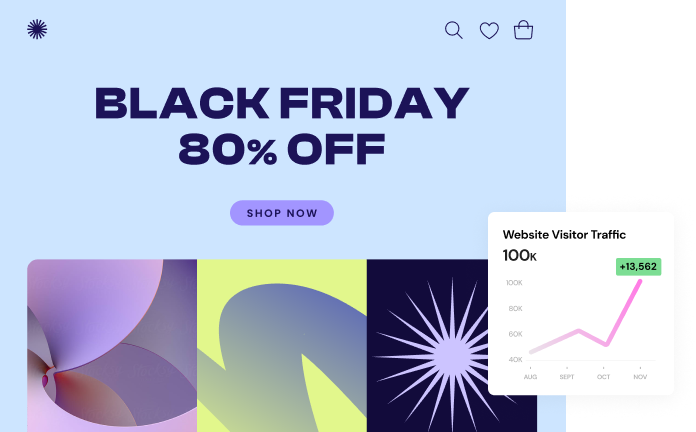
Top-Performing Website
- Super-Fast Websites
- Enterprise-Grade Security
- Any Site, Every Business
- 24/7 Expert Service
- Drag & Drop Website Builder, No Code Required
- Over 100 Widgets, for Every Purpose
- Professional Design Features for Pixel Perfect Design
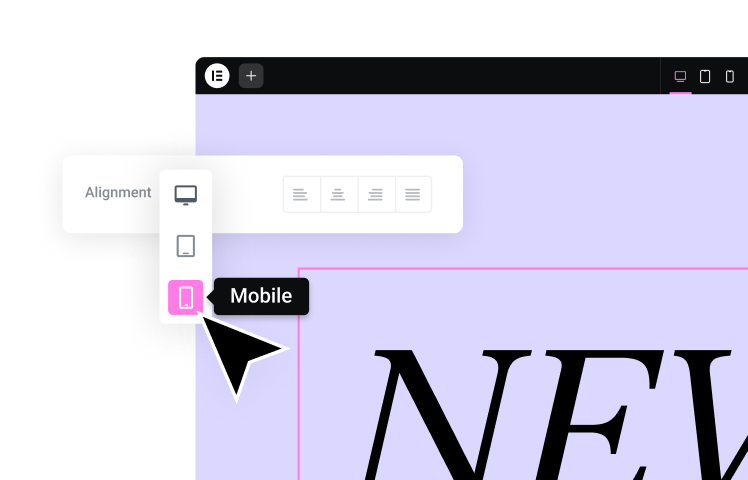
- Drag & Drop Website Builder, No Code Required
- Over 100 Widgets, for Every Purpose
- Professional Design Features for Pixel Perfect Design
- Marketing & eCommerce Features to Increase Conversion
- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking
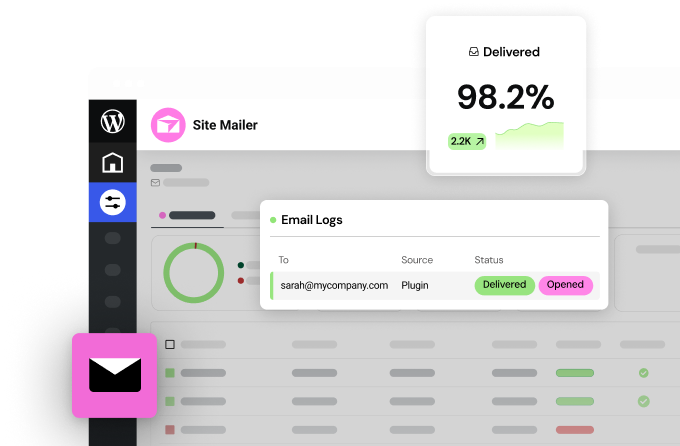
- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking
Mastering Background Image Sizing
The background-size Property
The background-size property controls how a background image is scaled to fit within its container. Here are the most common values:
- cover: The image is scaled to cover the entire container, maintaining its aspect ratio. Parts of the image may be cropped to ensure a full fit.
- contain: The image is scaled to fit fully inside the container, maintaining its aspect ratio. This might leave space around the image if the container’s aspect ratio differs.
- length: You can specify an explicit width and height (e.g., background-size: 200px 150px;).
- percentage: Sizes the image relative to its container’s width or height (e.g., background-size: 50% auto;).
Example:
CSS
body {
background-image: url('landscape. jpg');
background-size: cover;
}
Responsive Background Images
In modern web design, responsiveness is essential. Here’s how to make sure background images adapt well across different screen sizes:
- Flexible Units: Use percentage or viewport units (vw, vh) within the background-size property to allow the image to scale fluidly.
- Media Queries: Employ CSS media queries to apply different background-size values ( or even different background images) based on screen size or device orientation.
Example (Media Query):
CSS
@media (max-width: 768px) {
body {
background-image: url('landscape-mobile.jpg');
background-size: contain;
}
}
Precise Background Image Positioning
The background-position Property
The background-position property gives you granular control over where a background image is placed within its container. It accepts values in various formats:
Keywords
- top, bottom, left, right, center (positions the image relative to the edges)
- Combinations are allowed (e.g., top right)
Lengths
Pixel values (e.g., background-position: 20px 10px;) or viewport units for responsive positioning.
Percentages
Position the image as a percentage relative to the container’s width and height (e.g., background-position: 30% 75%;)
Example:
CSS
div {
background-image: url('texture.png');
background-position: center bottom;
}
Combining Positions
You can combine keyword, percentage, and pixel values in the background-position property to create complex and precise image placement within your design.
Example:
CSS
div {
background-image: url('icon.png');
background-repeat: no-repeat;
background-position: right 20px bottom 10px;
}
This would place an icon 20 pixels from the right edge and 10 pixels from the bottom edge of the container element.
Important: When using two values, the first value represents the horizontal position, and the second value represents the vertical position.
Fixed vs. Scrolling Backgrounds
The background-attachment Property
The background-attachment property controls how a background image behaves when the user scrolls the page. Here are the main values:
- scroll: The background image scrolls along with the content of the element (this is the default behavior).
- fixed: The background image remains fixed in its position relative to the viewport, creating the illusion that it stays in place while the content scrolls over it.
- local The background image scrolls along with the content of the element itself, even if that element has a scrolling mechanism.
The Parallax Effect
Parallax scrolling is a popular technique in which background images move at a slower rate than foreground content, creating an illusion of depth. This is achieved by using background-attachment: fixed, along with some JavaScript to adjust the background image’s position based on the scroll progress.
Design Considerations
When deciding between fixed and scrolling backgrounds, consider these factors:
- Visual Impact: Fixed backgrounds can create a dramatic or immersive effect, often used in hero sections.
- Readability: Ensure any text overlaid on a fixed background has sufficient contrast to remain legible.
- Performance: Fixed backgrounds, especially those used for parallax effects, can have a slight impact on performance. Use them strategically.
- User Experience: Avoid overusing fixed backgrounds or overly complex parallax, as these can distract or disorient users on some devices.
Advanced Background Image Techniques
Multiple Background Images
CSS allows you to layer multiple background images on a single element, creating rich visual effects with depth and complexity. Here’s how to achieve this:
- Comma-separated background-image values: List multiple url() values within the background-image property, separated by commas.
- Layer stacking order: The first image in the list is the bottom-most layer; subsequent images are stacked on top.
Example:
CSS
div {
background-image: url('gradient.png'), url('texture.jpg'), url('logo.svg');
background-repeat: no-repeat, repeat, no-repeat;
background-position: center, 0 0, right bottom;
}
Important: You can control the background-repeat,background-position, and other properties for each background image individually by supplying comma-separated lists for those properties as well.
Linear Gradients
Gradients are smooth transitions between two or more colors. The linear-gradient() function lets you create linear gradients for dynamic background effects.
Basic Syntax
CSS
background-image: linear-gradient(direction, color-stop1, color-stop2, …);
- direction: This sets the angle of the gradient. It can be expressed with keywords (to top, to bottom right) or numeric degrees (e.g., 45deg).
- color-stop: A color and its position along the gradient line (e.g., blue 0%, red 50%, green 100%)
Example:
CSS
body {
background-image: linear-gradient(to right, #f00 0%, #00f 100%);
}
Radial Gradients
Radial gradients create a color transition that radiates outward from a central point. Here’s how to use the radial-gradient() function in CSS:
Basic Syntax
CSS
background-image: radial-gradient(shape size at position, color-stop1, color-stop2, ...);
Shape
Defines the shape of the gradient. Can be:
- ellipse (default)
- circle
Size
Determines the extent of the gradient. Options include:
- closest-side, farthest-side, closest-corner, farthest-corner
- Explicit lengths or percentages.
Position
Sets the center point of the gradient. Uses a similar syntax to background-position.
Color-stop
Same as in linear gradients.
Example:
CSS
div {
background-image: radial-gradient(circle at center, #ff0000 0%, #0000ff 60%, #000 100%);
}
Tips
- Radial gradients can start from different positions within the container, not just the center. Experiment with the position value for interesting effects.
- Like their linear counterparts, radial gradients can add a sense of depth or visual focus to your background images.
Transparency with RGBA and Opacity
Let’s delve into how you can control transparency and create semi-transparent background effects:
RGBA Colors: RGBA extends the RGB color model by adding an alpha channel (A), which controls opacity. Values range from 0 (fully transparent) to 1 (fully opaque).
Example:
CSS
div {
background-color: rgba(255, 0, 0, 0.5); /* Half-transparent red */
}
Opacity Property: This property applies opacity to an entire element, including its background image. Values range from 0 to 1.
Example:
CSS
img {
opacity: 0.8; /* Makes the image 80% opaque */
}
Optimizing Background Images for Performance
Image Compression
Compressing images reduces their file size without excessively sacrificing quality. It’s a balancing act, and here are some tools and approaches to consider:
Image Optimization Tools
- Online services: TinyPNG, Squoosh, Kraken.io, and many others offer online tools.
- Software: Photoshop, GIMP, or dedicated image optimization software like ImageOptim.
- Elementor Image Optimizer: If you plan to mention Elementor, briefly introduce its built-in image optimization tool for a streamlined workflow.
Lossy vs. Lossless Compression
- Lossy: This reduces file size more significantly by permanently discarding some image data (suitable for photos; use judiciously).
- Lossless: Optimizes file size without any loss of quality (ideal for graphics and logos).
Finding the Sweet Spot: Experiment with different compression levels to find the best trade-off between file size and visual quality for your background images.
Preloading
Preloading hints to the browser that background images are important and should be fetched early in the page loading process. Here’s how to implement it using HTML’s <link> tag:
HTML
<link rel="preload" as="image" href="hero-image.jpg">
Optimize Background Images for Faster Loading Times
While background images can greatly enhance the visual appeal of your design, they can also impact page load speed if not optimized. By using an image optimizer plugin, you can compress background images to reduce their file size without sacrificing quality. This quick step ensures your page loads faster, improving user experience and helping with SEO. For best results, always aim to use optimized images alongside efficient CSS to strike the perfect balance between aesthetics and performance.
Background Images and Web Design Trends
Current Trends
Web design is constantly evolving, and background image usage naturally reflects these shifts. Here are a few notable trends:
- Bold Hero Images: Large, high-quality background images in hero sections are a mainstay. They set the mood for the site and capture visitor attention.
- Textures and Gradients: These add depth and interest without overwhelming the overall design. Gradients offer smooth color transitions, while textures bring a tactile quality.
- Illustrations as Backgrounds: Custom illustrations offer personality and uniqueness, especially when paired with bold typography.
- Overlays with Color and Opacity: Placing a colored overlay with transparency over a background image can create striking visual effects and improve text readability.
- Asymmetrical Layouts: Background images are powerful tools for emphasizing asymmetry in designs, creating a more dynamic and unexpected composition.
- Animation and Interaction: CSS animations or hover effects on background images add a touch of engagement and playfulness.
Accessibility Considerations
Remember, design trends shouldn’t come at the cost of accessibility! Here are key points to keep in mind:
- Contrast: Always ensure sufficient color contrast between any text overlayed on your background image and the image itself.
- Alternative Information: For background images that convey important content, offer text-based alternatives or use ARIA attributes to provide descriptions for screen readers.
- Busy Backgrounds: Complex images can be distracting for some users. If in doubt, opt for a simpler background to prioritize readability.
Let’s do that! Here are a few more techniques to improve your background image game.
Beyond the Basics
Background Blending Modes
CSS background blend modes determine how a background image interacts with the existing colors or content behind it. This opens up a whole range of creative possibilities. Some of the most common blending modes include:
- multiply: Darkens the result by multiplying the background and foreground colors.
- screen: Lightens the result by inverting, multiplying, and inverting again.
- overlay: Combines multiply and screen for a contrast effect.
- color-dodge: Brightens the background based on the foreground color.
- color-burn: Darkens the background based on the foreground color.
Example:
CSS
div {
background-image: url('image.jpg');
background-blend-mode: multiply;
}
Tips
- Experiment with different blend modes to achieve unique visual effects.
- Blending modes work best when the background image has areas of transparency.
- Be mindful of the readability of any text placed over images with blend modes applied.
Background Images with CSS Animations and Transitions
Adding CSS animations or transitions allows you to create dynamic background images that change over time. Here are a few ideas:
- Hover Effects: Change the background-size, background-position, or apply filters upon hovering over an element with a background image.
- Ken Burns Effect: Simulate the panning and zooming effect common in documentaries by slowly changing background-size and background-position.
- Animated Gradients: Cycle through color stops in a gradient using CSS animations for a mesmerizing transition effect.
Note: Use animations tastefully! Overly distracting animations can negatively impact the user experience.
Background Images as Sprites
Traditionally, an image sprite combines multiple smaller images into a single file, improving performance by reducing HTTP requests. This same technique can be applied to background images! Here’s how it works:
- Combine images: Create a sprite sheet containing all your small background images (e.g., icons).
- Use background-position: Precisely position each icon within the container element using background-position.
When to use this: It’s helpful when working with multiple small icons that are frequently used throughout your site.
Conclusion
Background images, when used thoughtfully, can transform a website’s look, feel, and overall user experience. By understanding the core CSS properties, exploring advanced techniques, and prioritizing optimization, you can create stunning visuals that don’t compromise performance.
Remember these key takeaways:
- Based on your image content, choose the right image formats (JPEG, PNG, GIF, SVG, WebP).
- Control image repetition, sizing, and positioning with precision.
- Experiment with fixed vs. scrolling backgrounds for unique design effects.
- Layer images, use gradients, and control transparency for creative depth.
- Optimize, pre-load, and potentially lazy load images to ensure lightning-fast loading times.
- Stay aware of current web design trends and accessibility guidelines.
Tools like Elementor can significantly simplify background image management, allowing you to focus on your design vision while performance optimizations are handled behind the scenes.
Whether you’re a seasoned web developer or just starting, background images are an essential tool in your web design toolkit. Don’t be afraid to experiment and push the boundaries of what’s possible!
Looking for fresh content?
By entering your email, you agree to receive Elementor emails, including marketing emails,
and agree to our Terms & Conditions and Privacy Policy.