What Exactly Are CSS Selectors?
Think of CSS selectors as the precise targeting system for your website’s styles. They’re the patterns you write in your stylesheet that tell the browser, “Hey, find these specific elements on the page and apply these styles to them.”
Grow Your Sales
- Incredibly Fast Store
- Sales Optimization
- Enterprise-Grade Security
- 24/7 Expert Service
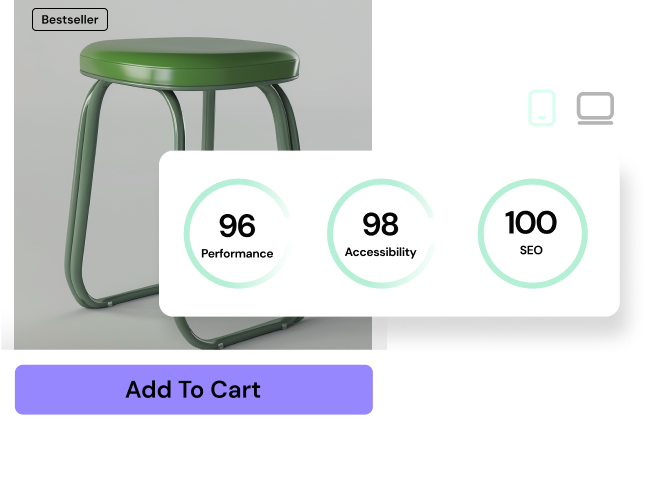
- Incredibly Fast Store
- Sales Optimization
- Enterprise-Grade Security
- 24/7 Expert Service
- Prompt your Code & Add Custom Code, HTML, or CSS with ease
- Generate or edit with AI for Tailored Images
- Use Copilot for predictive stylized container layouts

- Prompt your Code & Add Custom Code, HTML, or CSS with ease
- Generate or edit with AI for Tailored Images
- Use Copilot for predictive stylized container layouts
- Craft or Translate Content at Lightning Speed
Top-Performing Website
- Super-Fast Websites
- Enterprise-Grade Security
- Any Site, Every Business
- 24/7 Expert Service

Top-Performing Website
- Super-Fast Websites
- Enterprise-Grade Security
- Any Site, Every Business
- 24/7 Expert Service
- Drag & Drop Website Builder, No Code Required
- Over 100 Widgets, for Every Purpose
- Professional Design Features for Pixel Perfect Design
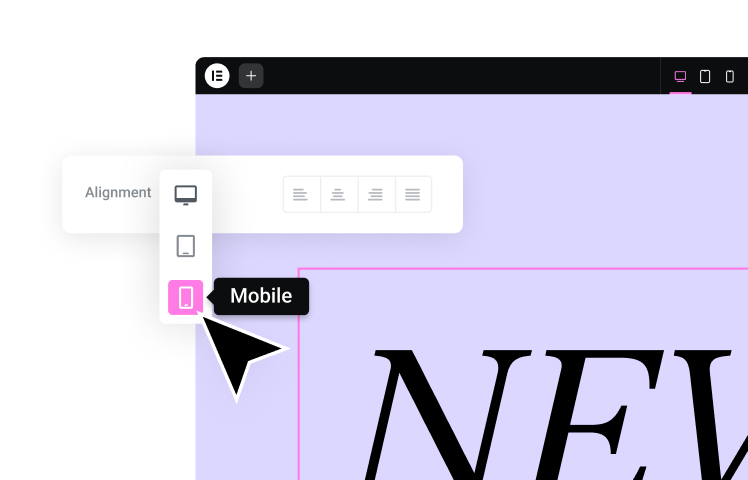
- Drag & Drop Website Builder, No Code Required
- Over 100 Widgets, for Every Purpose
- Professional Design Features for Pixel Perfect Design
- Marketing & eCommerce Features to Increase Conversion
- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking

- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking
Why Should You Care About Selectors?
In a nutshell, selectors give you laser-focused control over your design. Instead of applying styles to every element of a certain type (like all paragraphs), you can zero in on just the ones you want to change. This not only makes your code more efficient but also opens up a world of creative possibilities.
Imagine being able to style the first paragraph of each blog post differently, change the color of a button when someone hovers over it, or even add a cool effect to the first letter of every heading. All of this is possible with the right selectors in your toolkit.
And here’s the best part: mastering CSS selectors is more manageable than it might seem. We’ll guide you through everything you need to know and even show you how Elementor, a powerful website builder, can make the process even smoother.
Get ready to level up your CSS game and unlock a new level of design precision!
Understanding the Basics: Your Core Selector Toolkit
Let’s dive into the fundamental building blocks of CSS selectors – the ones you’ll use day in and day out to style your web pages. Think of them as the essential tools in your CSS toolbox.
Universal Selector (*)
The universal selector is like the wildcard of CSS. It matches every single element on your page. Want to apply a basic font to all your text? The universal selector has your back:
* {
font-family: sans-serif;
}
But be careful! Using the universal selector can lead to performance issues and make your CSS easier to maintain. It’s best used sparingly for very broad styling rules.
Element (Type) Selectors
These selectors target elements based on their HTML tag names. Want to style all your paragraphs? Use the p selector:
p {
line-height: 1.6;
}
This is a great way to set default styles for common elements, but it could be more specific. If you want more control, you’ll need to get more granular.
Class Selectors
Class selectors are where the real power of CSS begins. You can assign classes to HTML elements using the class attribute and then style those elements using a dot (.) followed by the class name:
<p class="intro">Welcome to my website!</p>
.intro {
font-size: 1.2em;
font-weight: bold;
}
This allows you to style multiple elements with the same class or even apply multiple classes to a single element for even more flexibility.
ID Selectors
ID selectors are similar to class selectors, but they’re even more specific. Each element can only have one ID, and you style them using a hash (#) followed by the ID name:
<h1 id="main-heading">This is my main heading</h1>
#main-heading {
color: #333;
text-align: center;
}
ID selectors are perfect for styling unique elements on your page, like headers, footers, or main content areas.
The Power of Specificity
Now that you know the basic selector types, it’s time to understand how browsers decide which styles to apply when multiple rules target the same element. This is where specificity comes in.
In a nutshell, specificity is a ranking system for CSS rules. The more specific a rule is, the higher its priority. Browsers will always apply the most specific rule that matches an element.
We’ll dive deeper into specificity in a later section, but for now, here’s a simple way to think about it:
- ID selectors are the most specific.
- Class selectors are less specific than ID selectors.
- Element selectors are the least specific.
If you have two rules that target the same element, the one with the higher specificity will win.
Attribute Selectors: Targeting Elements with Precision
Attribute selectors are like adding a laser sight to your CSS rifle. Instead of targeting elements solely based on their type or class, attribute selectors let you zero in on elements that have specific attributes and even specific values within those attributes.
Syntax and Examples
The basic syntax of an attribute selector looks like this:
element[attribute] { /* styles */ }
This selects all elements of the specified type that have the named attribute, regardless of its value.
For instance, to style all links (<a> elements) on your page, you could use:
a[href] {
color: blue;
text-decoration: underline;
}
To get even more specific, you can select elements with a particular attribute value:
input[type="submit"] {
background-color: #4CAF50;
color: white;
padding: 10px 20px;
}
This rule would only apply to input elements whose type attribute is set to “submit”.
Beyond Exact Matches
Attribute selectors offer even more flexibility with these additional options:
- Substring matching: [attribute*=”value”] selects elements whose attribute value contains the specified substring.
- Starts with: [attribute^=”value”] selects elements whose attribute value starts with the specified string.
- Ends with: [attribute$=”value”] selects elements whose attribute value ends with the specified string.
- Space-separated list: [attribute~=”value”] selects elements whose attribute value is a space-separated list that includes the specified value.
- Case-insensitivity: To make the match case-insensitive, you can use the i flag before the closing bracket (e.g., [attribute*=”value” i]).
Real-World Applications
Attribute selectors open up a wide range of possibilities:
Styling links differently based on their target:
CSS
a[target="_blank"] { /* Styles for links opening in a new tab */ }
- Use code with caution.
content_copy
Highlighting required form fields:
input[required] { /* Styles for required inputs */ }
- Use code with caution.
content_copy
Customizing elements based on data attributes:
div[data-role="banner"] { /* Styles for a banner div */ }
- Use code with caution.
content_copy
Elementor Integration
If you’re using Elementor, you can easily add attribute selectors to your stylesheets through the Custom CSS area. This gives you granular control over the look and feel of your website, even without touching the underlying HTML.
In the next section, we’ll explore the fascinating world of pseudo-classes and pseudo-elements, which take CSS selectors to the next level.
Pseudo-Classes and Pseudo-Elements: The Dynamic Duo of CSS Styling
Imagine being able to style elements not just based on their inherent properties but also based on their state (like when a button hovers over) or even parts of them that don’t exist in the HTML (like the space before the first letter of a paragraph). This is the magic of pseudo-classes and pseudo-elements.
Pseudo-Classes: Styling by State
Pseudo-classes are keywords you add to selectors to target elements that are in a specific state. They always start with a colon (:) and are incredibly versatile.
Interactive States
- :hover: Styles an element when the user’s mouse hovers over it.
- :active: Styles an element while it’s being activated (e.g., a button is clicked).
- :focus: Styles an element when it has keyboard focus (e.g., an input field).
Let’s create a stylish button that changes color when hovered over and clicked:
.button {
background-color: #007bff;
color: white;
padding: 10px 20px;
}.button:hover {
background-color: #0069d9;
}
.button:active {
background-color: #0062cc;
}
Structural Pseudo-Classes
These selectors target elements based on their position within the document structure.
- :first-child: Selects the first child element of its parent.
- :last-child: Selects the last child element of its parent.
- :nth-child(n): Selects every nth child element of its parent (e.g., :nth-child(2) selects every second child).
- :nth-last-child(n): Similar to :nth-child, but counting from the end.
- :only-child: Selects an element that is the only child of its parent.
Use these to create zebra-striped tables or style navigation menus:
li:nth-child(even) {
background-color: #f2f2f2;
}
Other Useful Pseudo-Classes
- :empty: Selects elements that have no children (including text nodes).
- :target: Selects the element whose ID matches the URL fragment identifier (e.g., #section2).
- :lang(language): Selects elements that have a specific language declaration (e.g., :lang(en) for English).
Pseudo-Elements: Styling the Unseen
Pseudo-elements are similar to pseudo-classes, but instead of targeting states, they target specific parts of an element that aren’t explicitly defined in the HTML. They start with two colons (::).
- ::before: Inserts content before an element’s content.
- ::after: Inserts content after an element’s content.
- ::first-letter: Styles the first letter of an element’s text.
- ::first-line: Styles the first line of an element’s text.
Let’s add a decorative icon before a list item:
li::before {
content: "\2713"; /* Unicode character for a checkmark */
margin-right: 5px;
}
Or style the first letter of a paragraph more artistically:
p::first-letter {
font-size: 2em;
float: left;
margin-right: 5px;
}
Elementor Pro Tip: You can leverage pseudo-elements in Elementor’s Theme Builder to create custom headers, footers, and other sections with advanced styling options.
Stay tuned for the next section, where we’ll explore how to combine selectors using the power of combinators.
Combinators: Linking Selectors to Create Powerful Relationships
Imagine you want to style a specific list item only when it’s nested within a specific unordered list. Or you may want to style a paragraph differently when it directly follows an image. This is where combinators swoop in to save the day. Combinators are special characters that you place between selectors to create relationships between elements, enabling you to target elements with surgical precision.
Descendant Combinator (Space)
The descendant combinator, represented by a single space, selects all descendants (children, grandchildren, great-grandchildren, and so on) of an element.
ul li {
/* Styles all list items within any unordered list */
}
In this example, all <li> elements that are descendants of any <ul> element on the page will be styled.
Child Combinator (>)
The child combinator is more specific than the descendant combinator. It selects only an element’s direct children.
ul > li {
/* Styles only the direct child list items of an unordered list */
}
This rule would only style list items that are immediate children of the <ul>. List items nested deeper within other elements within the list wouldn’t be affected.
Adjacent Sibling Combinator (+)
The adjacent sibling combinator selects the element that immediately follows another element and shares the same parent.
h2 + p {
/* Styles the paragraph that immediately follows an h2 heading */
}
In this case, only the paragraph that comes right after a <h2> heading would be styled, not any subsequent paragraphs.
General Sibling Combinator (~)
The general sibling combinator is similar to the adjacent sibling combinator, but it selects all siblings of an element that follow it, not just the immediate sibling.
h2 ~ p {
/* Styles all paragraphs that follow an h2 heading within the same parent */
}
This would style all paragraphs after an <h2>, even if there are other elements between them.
Advanced Combinators
CSS also offers more advanced combinators like the negation pseudo-class (not()) and the :has() pseudo-class (if supported by your target browsers). These allow for even more complex and specific targeting of elements.
Elementor Integration
Elementor’s custom CSS feature lets you harness the power of combinators to create intricate styling rules. By combining different selectors and combinators, you can target very specific elements and achieve unique visual effects.
For instance, style a button differently when it appears within a specific section of your page. You could achieve this by combining an ID selector for the section with a descendant combinator and a class selector for the button:
#my-section .button {
/* Styles the button only when it's inside the section with the ID 'my-section' */
}
Troubleshooting and Best Practices: Mastering Selector Expertise
Like any powerful tool, CSS selectors can be finicky at times. Styles might apply differently than you expect, your code might become a tangled mess, or your website’s performance could take a hit. But fear not! With some troubleshooting know-how and a sprinkle of best practices, you’ll be a selector ninja in no time.
Common Selector Errors
Even seasoned developers need to correct mistakes. Here are some common culprits that can cause your CSS to misbehave:
- Typos: A single missing character, incorrect case, or misplaced space can throw off your entire selector. Always double-check your code!
- Incorrect Syntax: Forgetting a colon in a pseudo-class or using the wrong combinator can lead to unexpected results. Consult a reference if you need clarification.
- Specificity Conflicts: If multiple selectors target the same element with different styles, the most specific one wins. This can lead to confusion if you are not aware of the specificity hierarchy.
Debugging Like a Pro
Your browser’s developer tools are your best friend when it comes to CSS debugging. Open the inspector, select the element you’re having trouble with, and examine the “Styles” tab. You’ll see which CSS rules are being applied, their specificity, and whether any rules are being overridden.
Performance Considerations
While selectors are incredibly powerful, they can also impact your website’s performance if you’re not careful. Overly complex selectors, especially those that involve many nested elements or multiple attribute selectors, can slow down the rendering process.
Elementor can help you optimize your CSS delivery by minimizing and combining stylesheets, but it’s still a good practice to keep your selectors as simple and efficient as possible.
Best Practices for Selector Success
Here are a few tips to keep in mind when working with selectors:
- Be Specific, But Not Overly So: Use the most specific selector necessary to target the elements you want to style, but avoid creating overly complex selectors that are difficult to read and maintain.
- Choose Meaningful Names: Use class and ID names that describe the purpose or content of the elements they’re attached to. Avoid generic names like “red” or “big.”
- Keep Your Code Organized: Use comments to explain your selectors and group related styles together. This will make your code easier to understand and modify later.
- Leverage Preprocessors: CSS preprocessors like Sass or Less can help you write more maintainable CSS with features like variables, nesting, and mixins.
- Test, Test, Test: Always test your styles in different browsers and on different devices to ensure they look and behave as expected.
By following these best practices and utilizing Elementor’s intuitive interface, you’ll be well on your way to mastering CSS selectors and creating stunning websites with ease.
Empowering Design with Elementor AI
Welcome to the future of web design, where artificial intelligence (AI) isn’t just a buzzword but a powerful tool that can transform the way you create websites. Elementor AI is at the forefront of this revolution, offering a suite of intelligent features that streamline the design process and unlock new creative possibilities.
Elementor AI’s Role: Your Design Sidekick
Imagine having a design assistant who could generate code, suggest layouts, and even design entire web pages based on your preferences. That’s precisely what Elementor AI offers.
Code Generation: Whether you’re a beginner struggling with CSS syntax or an experienced developer looking to save time, Elementor AI can help. It can generate CSS code for specific styles, saving you from tedious manual work.
Layout Suggestions: Are you stuck in a design rut? Elementor AI can analyze your content and suggest visually appealing layouts that match your brand’s style. This can be a game-changer, especially when you’re facing a creative block.
Full Page Design: If you’re looking for a complete website overhaul, Elementor AI can design entire pages based on your input. You can provide a few keywords, a mood board, or even a reference website, and Elementor AI will generate a range of design options for you to choose from.
Elementor AI Copilot: Your Virtual Design Assistant
Think of Elementor AI Copilot as your personal design mentor. It observes your actions within the Elementor editor and provides contextually relevant suggestions.
Need help writing compelling text? Copilot can generate options for you. Do you need help finding the right image? Copilot can suggest relevant visuals from your media library or even generate unique images using AI.
The more you use Copilot, the more it learns about your preferences and style, making its suggestions increasingly personalized and valuable.
A Case Study: Transforming a Blog Layout with Elementor AI
Let’s say you’re a blogger who wants to refresh the look of your posts. Elementor AI can generate a variety of layout options, experiment with different font pairings, and even suggest engaging call-to-action buttons. Thanks to the intuitive drag-and-drop interface and the power of AI, all of this is possible without writing a single line of code.
Balancing Creativity and Automation: AI as Your Co-Pilot, Not Autopilot
Now, you might be thinking, “Does this mean AI is going to replace web designers?” Not at all! Elementor AI is designed to be a powerful assistant, not a substitute for your creativity. It’s about empowering you to bring your vision to life faster and more efficiently.
Think of it this way: AI can handle repetitive, time-consuming tasks, like generating code or suggesting basic layouts. This frees you up to focus on the big picture—the overall design, the user experience, and the unique elements that make your website truly stand out.
It’s a collaboration, a partnership. You provide the creative spark, the vision, and the direction. Elementor AI provides the tools and support to help you realize that vision with less effort and more confidence.
Who Can Benefit from Elementor AI?
Elementor AI is a versatile tool that can benefit a wide range of users:
- Beginners: If you’re new to web design, Elementor AI can be a lifesaver. It guides you through the process, offers suggestions, and helps you create professional-looking websites without needing to learn complex code.
- Experienced Designers: Even seasoned pros can benefit from Elementor AI’s time-saving features and creative prompts. It can help you streamline your workflow, explore new ideas, and push the boundaries of your designs.
- Businesses of All Sizes: Whether you’re a small startup or a large corporation, Elementor AI can help you establish a strong online presence quickly and effectively. It’s a cost-effective solution that delivers high-quality results.
Conclusion
Congratulations! You’ve now embarked on a journey to master CSS selectors. We’ve covered a lot of ground, from the fundamental building blocks to advanced techniques, and we’ve even explored how Elementor and AI are changing the game of web design.
Remember, CSS selectors are your key to unlocking precise styling and creative freedom on your website. They empower you to target specific elements, create interactive experiences, and craft visually stunning designs.
Feel free to experiment, practice, and push the boundaries of what’s possible. The more you use selectors, the more intuitive they’ll become. With the help of tools like Elementor and its AI-powered features, you’ll be surprised at how quickly you can transform your design ideas into reality.
So, go forth and create beautiful, functional, and engaging websites with the power of CSS selectors. Your web design adventures await!
Looking for fresh content?
By entering your email, you agree to receive Elementor emails, including marketing emails,
and agree to our Terms & Conditions and Privacy Policy.