Table of Contents
Understanding the Basics: The Anatomy of a Select Tag
Let’s start by dissecting the core elements that make up a select tag. At its heart, a select tag is like a container that holds a list of options for the user to choose from. This container is defined by an opening <select> tag and a closing </select> tag.
Within this container, you’ll find individual <option> tags. Each option tag represents a single choice in the dropdown list. The text content of the option tag is what the user sees in the list, and the value attribute is what gets submitted to the server when the form is submitted. Think of the value as the secret code behind the scenes.
<select>
<option value="apple">Apple</option>
<option value="banana">Banana</option>
<option value="orange">Orange</option>
</select>
In this example, if the user selects “Banana,” the value “banana” will be sent along with the form data.
Default Selection
You can pre-select an option for the user by adding the selected attribute to the desired <option> tag. This is helpful when you want to provide a default choice or pre-fill a form based on previous user input.
<select>
<option value="apple">Apple</option>
<option value="banana" selected>Banana</option>
<option value="orange">Orange</option>
</select>
Now, when the page loads, “Banana” will be automatically selected in the dropdown.
Let’s break down the key components:
- <select>: The container for the dropdown list.
- <option>: Each choice within the list.
- value: The data sent to the server when the form is submitted.
- Text Content: The visible text in the dropdown.
- selected: The attribute used to pre-select an option.
This basic structure forms the foundation of any select tag. In the next section, we’ll explore how to level up your dropdowns with additional attributes and features to create even more interactive and user-friendly experiences.
Grow Your Sales
- Incredibly Fast Store
- Sales Optimization
- Enterprise-Grade Security
- 24/7 Expert Service
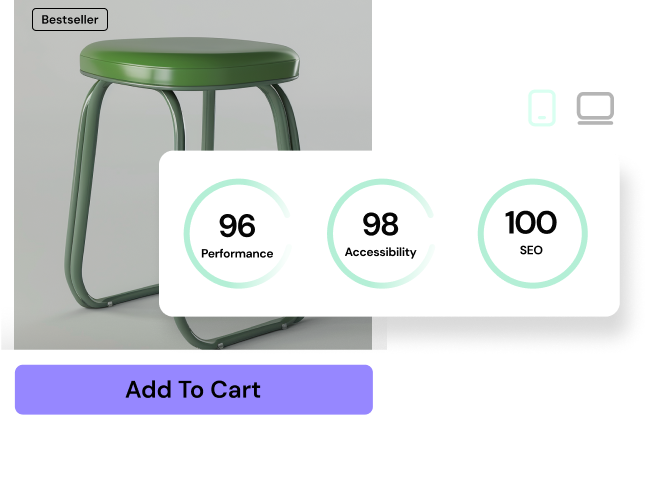
- Incredibly Fast Store
- Sales Optimization
- Enterprise-Grade Security
- 24/7 Expert Service
- Prompt your Code & Add Custom Code, HTML, or CSS with ease
- Generate or edit with AI for Tailored Images
- Use Copilot for predictive stylized container layouts
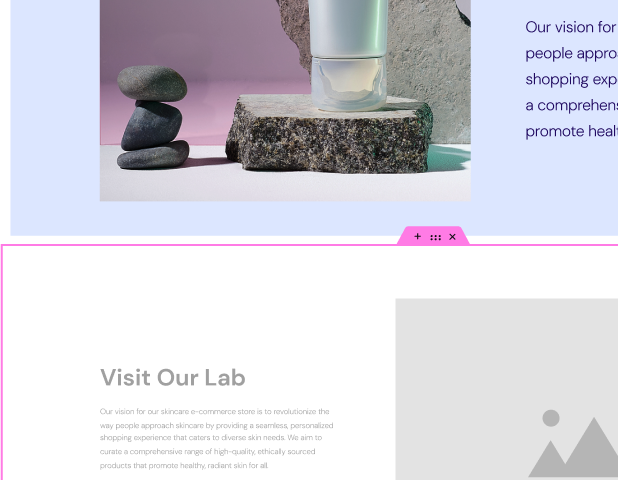
- Prompt your Code & Add Custom Code, HTML, or CSS with ease
- Generate or edit with AI for Tailored Images
- Use Copilot for predictive stylized container layouts
- Craft or Translate Content at Lightning Speed
Top-Performing Website
- Super-Fast Websites
- Enterprise-Grade Security
- Any Site, Every Business
- 24/7 Expert Service
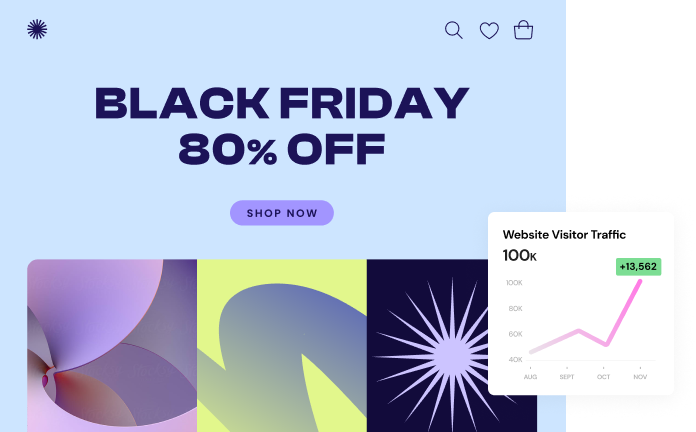
Top-Performing Website
- Super-Fast Websites
- Enterprise-Grade Security
- Any Site, Every Business
- 24/7 Expert Service
- Drag & Drop Website Builder, No Code Required
- Over 100 Widgets, for Every Purpose
- Professional Design Features for Pixel Perfect Design
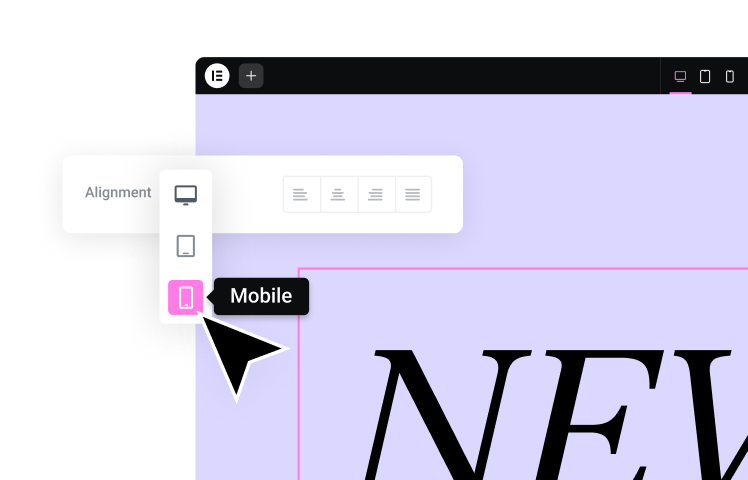
- Drag & Drop Website Builder, No Code Required
- Over 100 Widgets, for Every Purpose
- Professional Design Features for Pixel Perfect Design
- Marketing & eCommerce Features to Increase Conversion
- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking
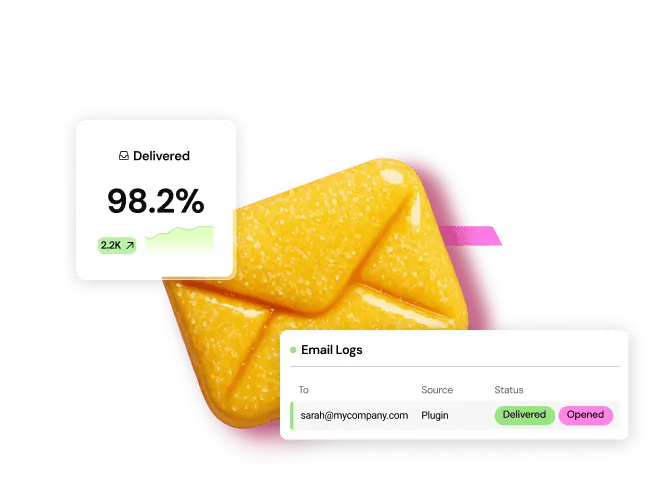
- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking
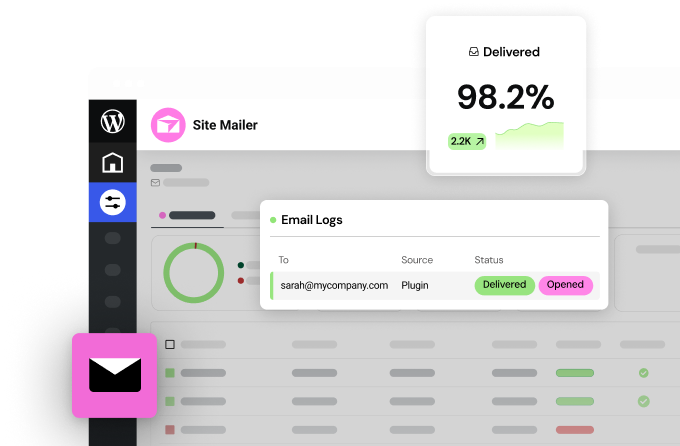
- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking
Leveling Up: Essential Attributes and Features
Now that we’ve covered the basic structure of a select tag let’s explore some powerful attributes and features that can take your dropdowns to the next level. These additions will help you create more versatile and user-friendly select elements that cater to different scenarios and preferences.
Multiple Selections: Empowering User Choice
The multiple attribute is your ticket, which allows users to select more than one option from your dropdown list. This is incredibly useful when you’re dealing with scenarios where multiple choices are valid, such as:
- Selecting multiple categories for a product
- Choosing multiple interests or hobbies
- Picking multiple recipients for an email
Adding multiple attribute is simple:
<select multiple>
<option value="apple">Apple</option>
<option value="banana">Banana</option>
<option value="orange">Orange</option>
</select>
With this attribute in place, users can hold down the Ctrl (or Cmd on Mac) key and click on multiple options to select them. The visual presentation may change slightly depending on the browser, but selected options will be highlighted or marked with checkboxes.
Disabled Options: Guiding User Choices
Sometimes, you’ll want to make certain options unavailable for selection. This could be because they’re temporarily out of stock, depend on other selections, or simply shouldn’t be chosen in the current context.
The disabled attribute comes to the rescue:
<select>
<option value="apple">Apple</option>
<option value="banana" disabled>Banana (Out of Stock)</option>
<option value="orange">Orange</option>
</select>
In this example, the “Banana” option will appear grayed out or visually distinct, and users won’t be able to select it. You can also add text to explain why the option is disabled, like “(Out of Stock)”.
By mastering these attributes, you can create more flexible and interactive forms that cater to a wider range of user needs. Up next, we’ll delve into the art of styling select tags with CSS to make them visually appealing and enhance your website’s overall design.
Styling with CSS: From Bland to Beautiful
Select tags aren’t the most visually appealing elements on a webpage out of the box. Their appearance can vary between browsers and operating systems, leading to consistency in your design. But fear not! With a sprinkle of CSS (Cascading Style Sheets), you can transform these plain dropdowns into stylish elements that complement your website’s aesthetic.
Basic Styling: A Touch of Personality
You can apply standard CSS properties to customize the look and feel of your select tags. Here are some common properties to play with:
- font-family, font-size, color: Change the font, size, and color of the text within the dropdown.
- background-color, border: Adjust the background color and border of the select element.
- padding: Add some breathing room around the text within the dropdown.
- width, height: Control the size of the dropdown container.
select {
font-family: sans-serif;
font-size: 16px;
color: #333;
background-color: #fff;
border: 1px solid #ccc;
padding: 10px;
width: 200px;
}
In addition to these basic properties, you can use CSS pseudo-classes to add interactive styling. For example, you can change the background color when the user hovers over the dropdown or when it’s in focus:
select:hover {
background-color: #f0f0f0;
}
select:focus {
border-color: #007bff; /* A nice blue highlight */
}
Advanced Styling: Unleash Your Creativity (and Some Limitations)
While you have some flexibility in styling select tags, there are limitations due to browser inconsistencies and the way these elements are rendered. You might not be able to fully customize the appearance of the dropdown arrow or the options themselves across all browsers.
However, there are workarounds and techniques you can explore:
- Styling individual options: You can use the option:checked selector to style the currently selected option differently.
- Creating custom dropdown menus: With a bit of JavaScript and CSS trickery, you can create completely custom dropdown menus that look exactly the way you want.
JavaScript Interactivity: Taking Control of Your Dropdowns
While HTML and CSS provide the structure and style for your select tags, JavaScript is the key to unlocking their full interactive potential. With JavaScript, you can dynamically manipulate your dropdowns, respond to user actions, and create seamless experiences that enhance the usability of your forms.
DOM Manipulation: Reaching into the Structure
The first step to working with select tags in JavaScript is understanding the Document Object Model (DOM). The DOM represents your HTML document as a tree-like structure, where each element (including your select tag) is a node. You can use JavaScript to access, modify, and manipulate these nodes.
To get started, you’ll need to grab a reference for your selected element. You can do this using methods like getElementById, querySelector, or getElementsByTagName. Here’s h
how you would target a select tag with the ID “mySelect”:
const selectElement = document.getElementById("mySelect");
Once you have a reference to the select element, you can access its properties, such as its value (the currently selected option), its options (an array of all the options within the select tag), and its length (the number of options).
Selecting and Deselecting Options: Making Choices Programmatically
You can use JavaScript to select or deselect options programmatically within a select tag. This is useful when you want to pre-fill a form based on user data or change the selected option in response to some event.
To select an option, you can set its selected property to true. Here’s how you would select the “Banana” option in our previous example:
const bananaOption = selectElement.options[1]; // Get the second option (index 1)
bananaOption.selected = true;
In a multi-select dropdown (one with multiple attributes), you can select multiple options by setting their selected properties to true.
Dynamically Adding/Removing Options: Flexibility On the Fly
The true power of JavaScript shines when you need to modify your select tags on the fly. You can dynamically add new options, remove existing ones, or even completely rebuild the options list based on user interactions or data fetched from a server.
To add a new option, you first create an option element using a document.createElement(“option”). Then, you set its value and textContent properties and append it to the select element using appendChild():
const newOption = document.createElement("option");
newOption.value = "grape";
newOption.textContent = "Grape";
selectElement.appendChild(newOption);
To remove an option, you can use the remove() method on the option element itself:
const bananaOption = selectElement.options[1]; // Get the second option
bananaOption.remove();
This dynamic control opens up a world of possibilities. You can create interactive forms where the options available to the user change based on their previous selections or update the list of options with real-time data from a database.
Handling Change Events: Reacting to User Selections
One of the most common interactions with a select tag is the change event. This event fires whenever the user selects a different option from the dropdown. You can use JavaScript to listen to this event and trigger actions based on the new selection.
Here’s how you can attach an event listener to the select tag:
selectElement.addEventListener("change", function() {
const selectedValue = selectElement.value;
// Do something with the selectedValue
});
Inside the event listener function, you can access the value property of the select element to get the value of the newly selected option. You can then use this value to update other parts of the page, make an AJAX request to fetch data or perform any other action you need.
Validation and Accessibility: Ensuring Usability for All
Your select tags might look sleek and function smoothly, but there are two crucial aspects you can’t afford to overlook: validation and accessibility. These elements ensure that your forms are user-friendly and usable by everyone, regardless of their abilities or the devices they use.
Validation: Keeping User Input in Check
It’s not uncommon for users to make mistakes or miss the required fields when filling out forms. That’s where validation comes in. Validation helps ensure that the data submitted through your select tags is accurate and complete.
The required Attribute
The simplest way to enforce selection in a select tag is by using the required attribute:
<select required>
</select>
When this attribute is present, the browser will prevent the form from being submitted if the user hasn’t selected an option from the dropdown.
Custom Validation with JavaScript
While the required attribute is handy, you might need more fine-grained control over your validation rules. For example, you might want to:
- Check if the selected value matches a specific pattern
- Ensure the user has selected a minimum or maximum number of options in a multi-select dropdown
- Validate the selected value against data fetched from a server
JavaScript empowers you to create custom validation logic tailored to your specific needs. You can listen for the change event on the select tag, check the selected value, and display error messages if the input doesn’t meet your criteria.
ARIA Attributes: Making Your Dropdowns Accessible
Accessibility is about ensuring that your website is usable by people with disabilities, including those who rely on screen readers or have difficulty using a mouse. For select tags, this means making sure they are navigable and understandable using keyboard commands and assistive technologies.
Accessible Rich Internet Applications (ARIA) attributes provide a way to enhance the accessibility of your select tags. Here are some key attributes to consider:
- role=”listbox”: Indicates that the element is a list box, which is how screen readers interpret select tags.
- aria-labelledby: Associates a label with the select tag, providing a descriptive name for screen reader users.
- aria-selected: Indicates which options are currently selected in a multi-select dropdown.
- aria-disabled: Indicates that an option is disabled and should not be selected.
By incorporating these ARIA attributes, you can ensure that your select tags are properly announced and interpreted by screen readers, making your forms accessible to a wider audience.
Keyboard Navigation: Empowering All Users
Keyboard navigation is essential for users who cannot use a mouse or prefer to interact with web pages using their keyboard. For select tags, this typically involves:
- Tabbing to the select tag to bring it into focus.
- Use the arrow keys to navigate through the options in the dropdown.
- Use the spacebar or enter key to select an option.
Most browsers natively support keyboard navigation for select tags. However, if you’re creating custom dropdown menus with JavaScript, you’ll need to implement the necessary keyboard event handlers to ensure a seamless experience for keyboard users.
Advanced Techniques and Best Practices: Taking Your Select Skills to the Next Level
Now that you’ve mastered the fundamentals of select tags let’s explore some advanced techniques and best practices that will elevate your forms and provide the best possible user experience.
The size Attribute: Displaying Multiple Options at Once
By default, a select tag only displays one option at a time, requiring the user to click or tap to expand the dropdown. However, you can change this behavior using the size attribute. This attribute determines how many options are visible in the dropdown list without having to expand it.
<select size="4">
<option value="apple">Apple</option>
<option value="banana">Banana</option>
<option value="orange">Orange</option>
<option value="grape">Grape</option>
<option value="mango">Mango</option>
</select>
In this example, the select tag will display four options at once, with a scrollbar appearing if there are more options than the specified size. This is particularly useful when you have a limited number of options and want to present them all without forcing the user to click.
The autofocus Attribute: Grabbing Attention
If you want to direct the user’s attention to a specific select tag on your page, you can use the autofocus attribute. This attribute automatically places the cursor in the select tag when the page loads, making it ready for interaction.
<select autofocus>
</select>
The catalyst Element: Autocomplete for the Win
Have you ever wished your select tags could offer autocompletion, similar to how search engines suggest results as you type? The HTML5 data list element allows you to do just that. A data list is a list of pre-defined options that are displayed to the user as they type into the select tag’s input field.
Here’s how you create and associate a data list with a select tag:
<datalist id="browsers">
<option value="Chrome">
<option value="Firefox">
<option value="Safari">
<option value="Edge">
</datalist>
<select list="browsers">
</select>
In this example, as the user starts typing into the select tag, the browser will display matching options from the “browsers” data list, making it easier for the user to find and select the desired option.
Cross-Browser Compatibility: Navigating the Quirks
While modern browsers generally handle select tags consistently, there can still be differences in how they render these elements. To ensure your dropdowns look and behave as expected across different browsers, consider the following tips:
- Normalize styles: Use a CSS reset or normalize stylesheet to minimize default browser styling differences.
- Test thoroughly: Check your select tags on various browsers (Chrome, Firefox, Safari, Edge) and operating systems (Windows, macOS, iOS, Android) to identify and fix any inconsistencies.
- Use vendor prefixes (if necessary): If you’re using newer CSS properties that might not be fully supported across all browsers, consider using vendor prefixes (e.g., -webkit- for Chrome and Safari).
By taking a proactive approach to cross-browser compatibility, you can create a more consistent and polished user experience.
Best Practices: Crafting User-Friendly Dropdowns
Beyond the technical details, there are several best practices you can follow to create select tags that are intuitive, accessible, and enjoyable to use:
- Use clear and concise labels: Make sure the purpose of the dropdown is obvious by using descriptive labels.
- Order options logically: If your options are in a natural order (e.g., alphabetical or numerical), arrange them accordingly.
- Limit the number of options: Long dropdowns can be overwhelming. If you have a large number of options, consider using a different input type (e.g., a search bar) or breaking the options into smaller groups.
- Provide a default option: If applicable, provide a default option (e.g., “Select an option”) to avoid confusion and ensure the user makes an intentional choice.
Adhering to these best practices allows you to create select tags that enhance user experience and contribute to your website’s overall usability.
Elementor AI Copilot and Website Builder: A Powerful Combo for Select Tags
While mastering the intricacies of the <select> tag is essential for any web developer, there’s no denying that manually coding and styling forms can be time-consuming. If you’re looking for a more streamlined and efficient approach, Elementor AI Copilot and the Elementor Website Builder offer a powerful solution.
Elementor Website Builder: Streamlining Select Tag Creation
Imagine creating visually stunning and interactive select tags without writing a single line of code. Elementor empowers you to do just that. Its intuitive drag-and-drop interface allows you to effortlessly add select elements to your web pages, customize their appearance, and even integrate them with dynamic content.
With Elementor, you can:
- Drag and drop select tags: Simply select the “Form” widget and add a “Select” field to your layout.
- Customize with ease: Style the dropdown using a variety of pre-designed templates or create your unique look with the visual editor.
- Connect with dynamic content: You can populate your select options from external sources like databases or APIs, making your forms more flexible and informative.
This streamlined approach not only saves time but also opens up the world of web design to those without extensive coding experience.
Elementor AI Copilot: Your Virtual Form Assistant
Now, imagine having an intelligent assistant by your side, guiding you through the process of creating and optimizing your select tags. Enter Elementor AI Copilot.
This AI-powered tool can:
- Generate code snippets: Need to add a specific behavior to your select tag? Elementor AI Copilot can write the code for you, saving you precious development time.
- Offer design suggestions: Need help with how to style your dropdown? Elementor AI Copilot can offer intelligent recommendations based on your website’s design and content.
- Provide context-aware assistance: Elementor AI Copilot learns from your actions and preferences, tailoring its suggestions to your specific needs.
By leveraging the power of AI, Elementor empowers you to create more sophisticated and user-friendly forms, even if you need to become a coding expert.
The combination of Elementor’s intuitive website builder and the intelligent assistance of Elementor AI Copilot is a game-changer for anyone working with select tags. Whether you’re a seasoned developer or a newcomer to web design, these tools can help you create visually stunning, interactive, and accessible forms that elevate the user experience on your website.
Conclusion
Congratulations! You’ve learned about the HTML select tag, from its basic anatomy to advanced techniques and best practices. You now understand how to create visually appealing, interactive, and accessible dropdowns that elevate the user experience on your websites.
Armed with this knowledge, you can confidently incorporate select tags into your forms, empowering users to make choices, filter content, and provide valuable input. Whether you’re building a simple contact form or a complex product selection interface, the select tag is a versatile tool that deserves a prominent place in your web development arsenal.
Remember, the key to mastering any skill is practice and experimentation. Feel free to try out different attributes, styling techniques, and JavaScript interactions. Embrace the power of the select tag, and watch as your forms come to life with interactive functionality.
As you continue your web development journey, consider exploring tools like Elementor Website Builder and Elementor AI Copilot. They can streamline your workflow, provide intelligent assistance, and unlock new possibilities for creating stunning and user-friendly websites.
Looking for fresh content?
By entering your email, you agree to receive Elementor emails, including marketing emails,
and agree to our Terms & Conditions and Privacy Policy.