Table of Contents
Comments enhance the readability, organization, and long-term maintainability of your CSS projects. Whether you’re working solo or collaborating with a team, well-placed comments can streamline your workflow and help you (or others) understand your code months or even years down the line.
In this guide, we’ll dive into the world of CSS comments, covering everything from basic syntax to clever ways to use them for debugging, collaboration, and future planning. We’ll even explore how website builders like Elementor and optimized hosting solutions like Elementor Hosting can enhance your commenting experience and boost your site’s performance.
CSS Comment Basics
The Syntax
The foundation of CSS commenting lies in a simple syntax: /* your comment here */. The browser will ignore everything enclosed within those opening and closing symbols. Let’s break it down:
- Opening: The /* signals the start of a comment.
- Content: This is where you place your actual text, which can be anything you find helpful.
- Closing: The */ marks the end of your comment.
Single-Line Comments
Use single-line comments for brief notes or labeling specific CSS rules:
CSS
/* This is a single-line comment */
p {
color: blue; /* Sets the text color to blue */
}
Multi-Line Comments
For longer explanations, code blocks you want to disable temporarily, or detailed notes, multi-line comments are your friend:
CSS
/*
This is a multi-line comment.
It can span multiple lines for more
detailed explanations or descriptions.
*/
Important Note: You cannot nest comments within other comments in CSS. Attempting to do so might lead to unexpected rendering issues.
Examples
Seeing comments in action helps solidify your understanding. Here are a few common use cases:
Explaining a Choice:
CSS
h1 {
font-family: 'Arial', sans-serif; /* Using Arial for a clean, modern look */
}
Labeling Sections:
CSS
/* ------- Header Styles ------- */
header {
background-color: #f0f0f0;
}
Temporarily Disabling Code (Commenting Out):
CSS
/* p {
color: red;
} */
Adding Notes within Rules:
CSS
.button {
background-color: green; /* Primary action color */
padding: 10px 20px; /* Generous padding for readability */
}
Grow Your Sales
- Incredibly Fast Store
- Sales Optimization
- Enterprise-Grade Security
- 24/7 Expert Service
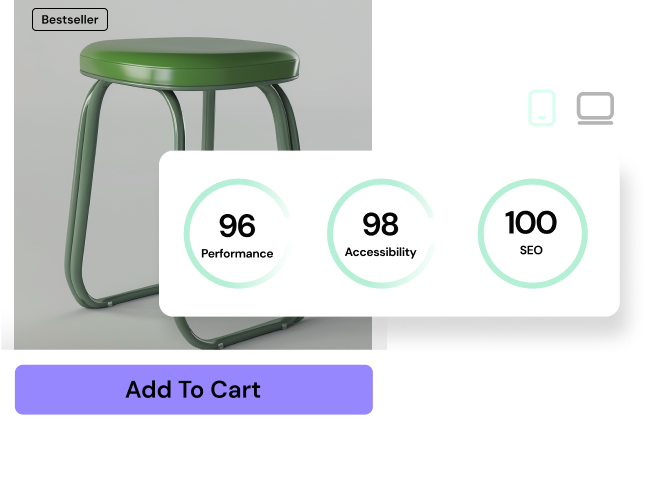
- Incredibly Fast Store
- Sales Optimization
- Enterprise-Grade Security
- 24/7 Expert Service
- Prompt your Code & Add Custom Code, HTML, or CSS with ease
- Generate or edit with AI for Tailored Images
- Use Copilot for predictive stylized container layouts

- Prompt your Code & Add Custom Code, HTML, or CSS with ease
- Generate or edit with AI for Tailored Images
- Use Copilot for predictive stylized container layouts
- Craft or Translate Content at Lightning Speed
Top-Performing Website
- Super-Fast Websites
- Enterprise-Grade Security
- Any Site, Every Business
- 24/7 Expert Service

Top-Performing Website
- Super-Fast Websites
- Enterprise-Grade Security
- Any Site, Every Business
- 24/7 Expert Service
- Drag & Drop Website Builder, No Code Required
- Over 100 Widgets, for Every Purpose
- Professional Design Features for Pixel Perfect Design
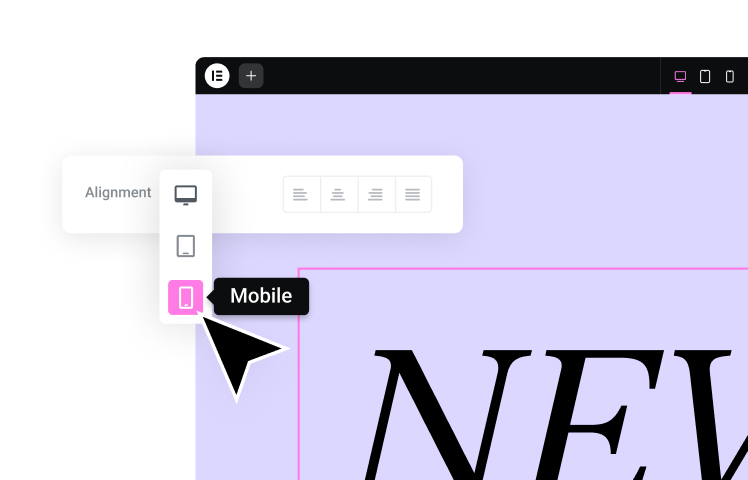
- Drag & Drop Website Builder, No Code Required
- Over 100 Widgets, for Every Purpose
- Professional Design Features for Pixel Perfect Design
- Marketing & eCommerce Features to Increase Conversion
- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking

- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking
Practical Uses of CSS Comments
Code Organization
Well-organized CSS is crucial for maintainability. Comments are your allies in achieving better structure:
- Sectioning: Clearly demarcate major sections of your stylesheet for easier navigation:
CSS
/* ------------------ Header Styles ------------------ */
/* ------------------ Main Content Styles ------------------ */
/* ------------------ Sidebar Styles ------------------ */
- Complex Selectors: Explain the purpose and logic behind intricate or unusual selectors:
CSS
nav ul li:nth-child(odd) a { /* Targets links within odd-numbered list items for styling */
background-color: #e8e8e8;
}
- Non-Obvious Techniques: If you employ a clever CSS trick, add a comment so you (or others) understand it later:
CSS
.clearfix::after { /* Uses the clearfix hack for reliable float clearing */
content: "";
display: table;
clear: both;
}
Debugging
When your CSS isn’t behaving as expected, comments help pinpoint the problem:
Isolating Issues: systematically comment out blocks of code. If the issue disappears, you’ve narrowed down the problem area.
CSS
/*
p {
color: red;
}
*/
Troubleshooting Notes: Add comments as you experiment, tracking what you’ve tried and the results, which will save you time in the long run.
CSS
p {
color: blue; /* Changed from red to test if the issue is related to color */
}
Future Debugging: If you find a temporary workaround, leave comments explaining the issue and your fix. This will help you address it properly later.
Collaboration
When working with others, comments are communication lifelines:
- Explaining Changes: When you modify CSS, leave comments outlining the reasons behind the change and any potential impact on other areas of the site.
- Sharing Design Decisions: Comments clarify the rationale behind styling choices, promoting consistency and understanding within your team
- “TODO” Reminders: Mark incomplete sections or planned enhancements with comments for yourself or collaborators.
CSS
/* TODO: Improve responsiveness of navigation menu on mobile devices */
Advanced Commenting Techniques
Commenting for Future Updates
Proactive commenting helps streamline future changes and updates:
Version Notes: Keep a commented changelog within your CSS, tracking modifications, dates, and who made the changes.
CSS
/* ------- Version History ------- */
/* 2024-03-15 (Jane Doe): Updated button colors for brand consistency */
/* 2024-02-28 (John Smith): Added hover effects to links */
Planned Changes: Mark areas where you intend to add functionality or make revisions, using comments as roadmaps for development.
CSS
/* Future Feature: Add animation to the image carousel */
Conditional Comments
Legacy Browser Hacks: While generally discouraged, if you must target specific older browsers, conditional comments were once the way to do it. However, modern CSS techniques make them largely obsolete.
Style Guides
If you work with a team, establishing commenting standards can significantly improve code consistency and maintainability. Consider these aspects:
- Formatting: Decide on single-line vs. multi-line usage conventions.
- Required Comments: Specify when comments are mandatory (e.g., major sections, complex rules).
- Example Templates: Provide comment templates to encourage clarity.
Commenting and Accessibility
While CSS comments aren’t directly visible to users, they can enhance the developer experience for maintaining accessible code:
Explaining ARIA attributes: If you use ARIA attributes for screen readers, add short comments explaining their purpose and intended effect.
HTML
<button aria-label="Close Menu" aria-expanded="false">
</button>
- Documenting Accessibility Fixes: When you address accessibility issues, comments provide context for why changes were made, aiding future maintenance.
- Accessibility Testing Notes: Use comments to log the results of accessibility audits or tests, guiding further improvement efforts.
Important: Remember, comments don’t replace proper semantic HTML and ARIA usage. They are a complementary tool for accessible development.
When NOT to Use CSS Comments
Over-Commenting
Finding balance is critical. While comments are helpful, too many can make your CSS cluttered and harder to read. Avoid these pitfalls:
- Redundancy: Don’t explain self-evident code (e.g., /* Color: blue */ next to a property that clearly sets a color).
- Excessive Detail: Aim for clarity and conciseness. Overly long-winded comments can be just as unhelpful as no comments at all.
Sensitive Information
- Security Risks: Never put passwords, API keys, or other sensitive data within CSS comments. Even if they’re not visible to users, they could be exposed if your files are compromised.
Alternatives to Comments
Sometimes, there are better ways to address certain needs:
- Self-Explanatory Code: Strive to write CSS with clear class names and selectors that minimize the need for excessive comments.
- External Documentation: For complex logic or design rationale, a separate documentation file might be more suitable than extensive comments within your CSS.
- Preprocessors: Tools like Sass or LESS offer features like variables and mixins, which can often replace the need for certain types of CSS comments.
Elementor Website Builder: Streamlining CSS Commenting
Visual Interface
Elementor’s intuitive drag-and-drop builder provides a user-friendly way to incorporate comments directly into your design workflow:
- Contextual Commenting: Add comments to specific elements or sections of your design, keeping your notes closely tied to the relevant visual components.
- Simplified Organization: Elementor’s interface may help visualize the structure of your code, making it easier to place comments strategically for improved organization.
Live Editing
As you make real-time adjustments to your website’s design, the ability to add and modify comments alongside offers several advantages:
- Iterative Refinement: Comment to explain the reasoning behind styling choices as you experiment and refine your design.
- Design History: Track your thought process over time, providing a valuable reference point if you need to revisit decisions later.
Collaboration Features
If you work within a team using Elementor, its collaboration tools might offer ways to enhance commenting practices:
- Shared Notes: Easily leave comments for other team members, keeping communication directly connected to the design itself.
- Revision History: Comments might integrate with Elementor’s revision history system, allowing you to track comments alongside visual changes to your website.
Important Note: The specific ways Elementor supports commenting depend on its features and how you integrate it into your workflow.
Elementor Hosting: Performance and Commenting
Optimized for Speed
Elementor Hosting is built on a robust infrastructure designed to deliver fast and reliable WordPress websites. This has a subtle but important impact on commenting:
- Reduced Impact on Load Time: With Elementor Hosting’s infrastructure (Google Cloud Platform, Cloudflare Enterprise CDN, etc.), the overhead of well-structured CSS comments is likely to be negligible.
- Freedom to Comment Thoroughly: You can focus on writing helpful comments with less concern over their effect on page speed.
Caching and Minification
Elementor Hosting includes built-in optimizations that work in tandem with your commenting practices:
- Caching: Caching mechanisms store pre-processed versions of your website, including CSS, reducing the need for the browser to parse comments repeatedly.
- Minification: Automatic minification removes whitespace and unnecessary characters from your CSS, including those within comments. This keeps your code compact without sacrificing the readability of your comments before minification.
- Advanced Optimizations: Depending on your Elementor Hosting plan, features like automatic CSS and JS file optimization and HTML minification further streamline code delivery.
With Elementor Hosting, you can optimize your website’s speed and utilize CSS comments effectively with the right strategies and tools.
Best Practices for CSS Commenting
Clarity and Conciseness
Aim for comments that are both informative and easy to digest. Here’s how:
- Plain Language: Avoid jargon or overly complex explanations. Write comments as if you’re explaining concepts to a fellow developer.
- Targeted Notes: Focus each comment on a specific concept, CSS rule, or code section. Avoid long, rambling comments.
- Examples: When appropriate, a quick example can illustrate a concept far better than a lengthy explanation.
Consistency
Establish a clear commenting style for yourself or your team:
- Formatting: Agree on single-line vs. multi-line usage conventions and preferred indentation.
- Templates: Create standard comment templates for common scenarios (e.g., explaining design choices, temporary fixes, licensing information)
- Style Guide: If working in a team setting, incorporate commenting guidelines into your project’s style guide. Elementor may have tools to help with this.
Commenting as a Learning Tool
Use comments to reinforce your own CSS knowledge and to help others learn:
- Explain Your Thought Process: When solving a CSS problem, comment on your steps, especially if you arrive at a non-obvious solution.
- Reference Resources: If you discover a technique online, add a comment with a link to the original source for future reference.
- Beginner-Friendliness: If you anticipate less experienced developers working with your code, include comments that explain basic CSS concepts.
The Future of CSS Commenting
Evolving Web Development Practices
As web development methodologies shift, so might the role of CSS comments:
- Component-Based Design: Reusable components could lead to less commenting within CSS files, as the logic might be encapsulated within component documentation.
- Increased Complexity: As new CSS features emerge, comments for advanced techniques like CSS Grids or complex animations might become even more important.
Preprocessors
The continued popularity of preprocessors (Sass, LESS) offers additional ways to integrate comments:
- Nested Comments: Preprocessors allow nested comments, enabling more structured commenting during development and then removing them for production.
- Advanced Comment Features: Preprocessors might offer their own syntax enhancements for commenting, like variables or mixins within comments.
Automation
- Comment Generation: Automated tools could help generate basic comments based on code structure, saving time on structural comments.
- Linting and Style Checking: Build processes that might enforce commenting rules, encouraging consistent practices within teams.
Important: The future of CSS commenting likely blends these trends with the enduring need for clear, well-structured code that’s easy to understand and maintain.
Conclusion
CSS comments, while often overlooked, are powerful allies in your web development journey. They offer a multitude of benefits, from streamlining organization and debugging to enhancing collaboration and future-proofing your projects.
Remember, the key lies in using comments strategically. Write clear, concise notes that genuinely add value to your codebase.
By integrating CSS commenting best practices into your workflow and leveraging the advantages of tools like Elementor Website Builder and Elementor Hosting, you’ll create well-structured, maintainable, and collaborative web projects.
Even seemingly small things like comments, when used thoughtfully, make a big difference in the long-term success of your web development endeavors!
Looking for fresh content?
By entering your email, you agree to receive Elementor emails, including marketing emails,
and agree to our Terms & Conditions and Privacy Policy.