Table of Contents
How HTML Handles Images
Before we discuss centering techniques, let’s familiarize ourselves with the basics of how images are incorporated into web pages using HTML.
The <img> Tag
At its core, the <img> tag is responsible for embedding images into your HTML document. It has the following essential attributes:
- src: This attribute specifies the image’s URL (Uniform Resource Locator), pointing the browser to where it can find the image file.
- alt: This attribute provides a text-based description of the image. It’s crucial for accessibility, as screen readers rely on this text to convey the image’s content to visually impaired users. Additionally, the alt text is displayed if the image fails to load for any reason.
Inline vs. Block Elements
HTML elements fall into two main categories:
- Inline Elements: These elements don’t start on a new line and only take up as much width as their content requires. Images, by default, are inline elements.
- Block-level Elements: These elements start on a new line and extend to the full width of their container. Common examples include paragraphs (<p>), headings (<h1>, <h2>, etc.), and divs (<div>). Understanding this distinction is key because certain centering techniques are specifically designed for block-level elements.
Grow Your Sales
- Incredibly Fast Store
- Sales Optimization
- Enterprise-Grade Security
- 24/7 Expert Service
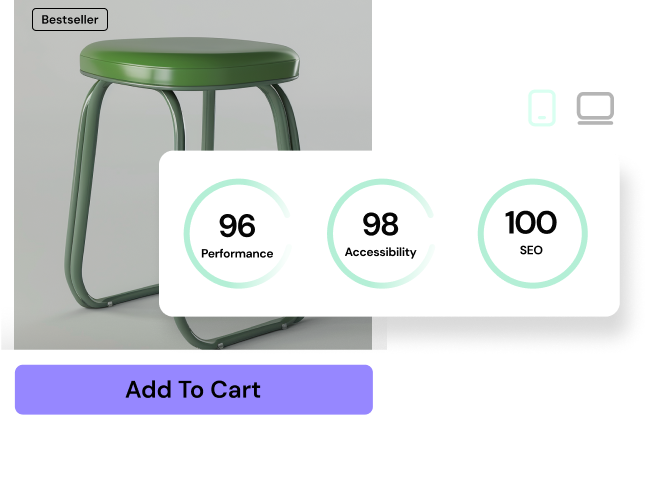
- Incredibly Fast Store
- Sales Optimization
- Enterprise-Grade Security
- 24/7 Expert Service
- Prompt your Code & Add Custom Code, HTML, or CSS with ease
- Generate or edit with AI for Tailored Images
- Use Copilot for predictive stylized container layouts
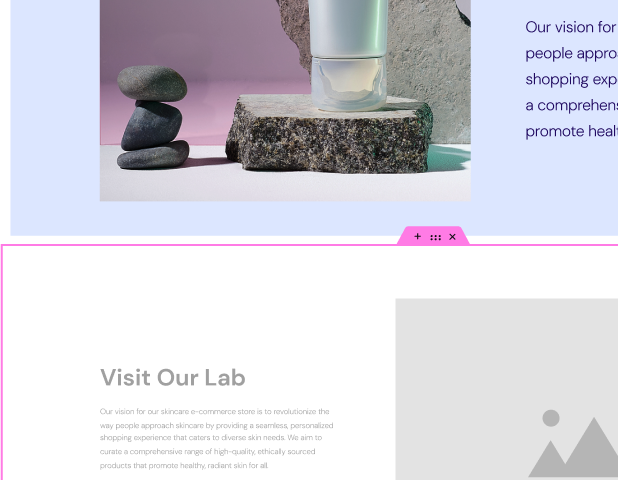
- Prompt your Code & Add Custom Code, HTML, or CSS with ease
- Generate or edit with AI for Tailored Images
- Use Copilot for predictive stylized container layouts
- Craft or Translate Content at Lightning Speed
Top-Performing Website
- Super-Fast Websites
- Enterprise-Grade Security
- Any Site, Every Business
- 24/7 Expert Service
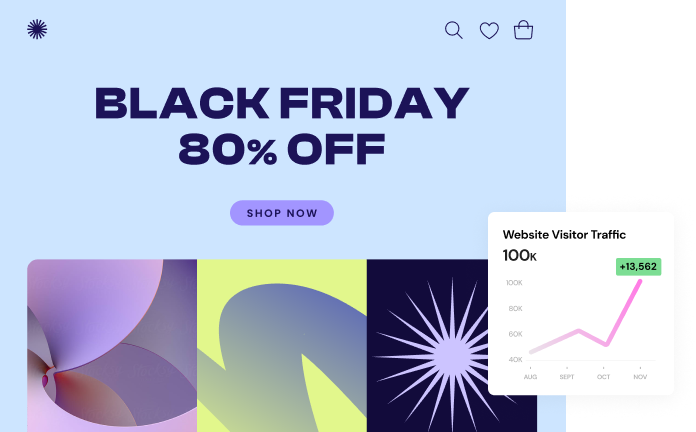
Top-Performing Website
- Super-Fast Websites
- Enterprise-Grade Security
- Any Site, Every Business
- 24/7 Expert Service
- Drag & Drop Website Builder, No Code Required
- Over 100 Widgets, for Every Purpose
- Professional Design Features for Pixel Perfect Design
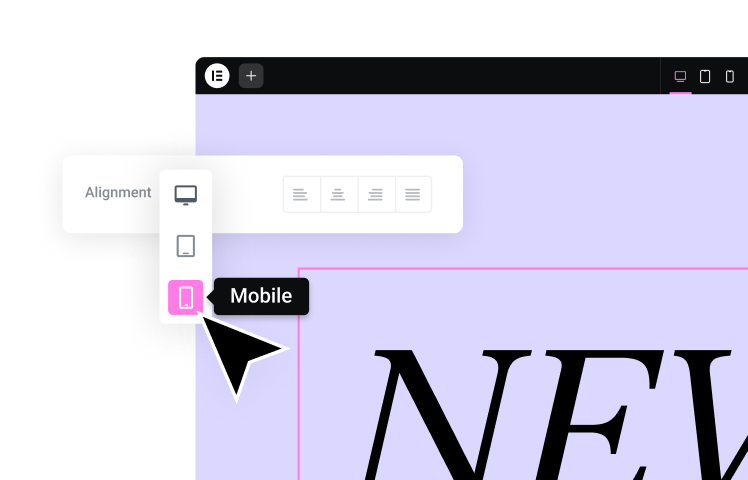
- Drag & Drop Website Builder, No Code Required
- Over 100 Widgets, for Every Purpose
- Professional Design Features for Pixel Perfect Design
- Marketing & eCommerce Features to Increase Conversion
- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking
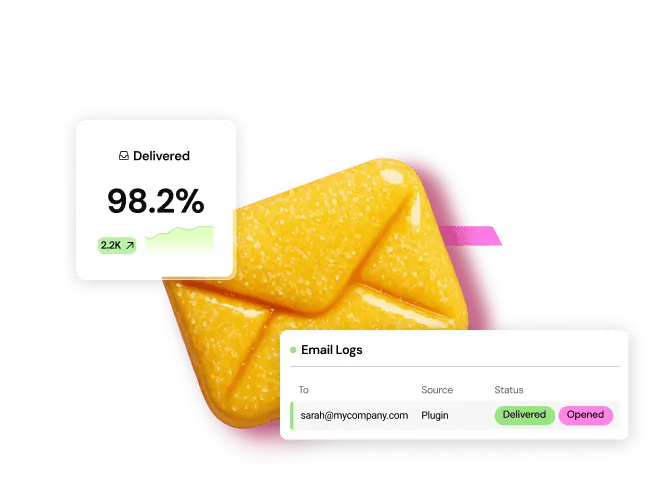
- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking
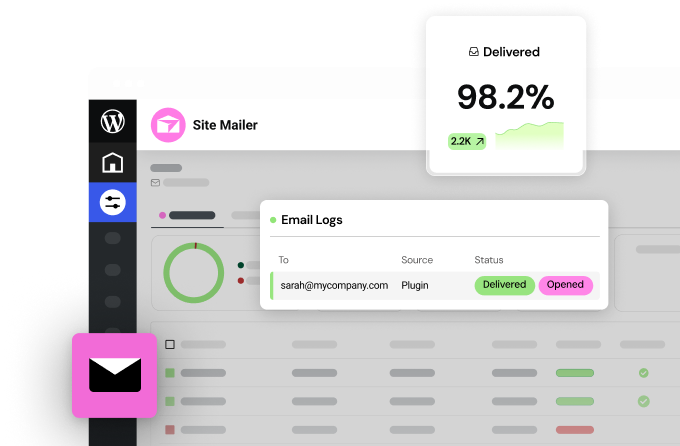
- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking
Centering Images with Traditional HTML/CSS Methods
Using text-align: center (for Inline Images)
One of the simplest ways to horizontally center an image is by using the CSS text-align property. Here’s how:
Wrap the Image in a Block-level Element
Since text-align primarily works on block-level elements, wrap your <img> tag within a <div> or a <p> tag.
Apply text-align: center
Add the following CSS to the block-level element containing your image:
HTML
/* Example using a <div> */
<div style="text-align: center;">
<img decoding="async" src="image-url.jpg" alt="Image Description">
</div>
Explanation: The text-align: center property instructs the browser to center all inline content within the specified block-level element, including your image.
Limitations: While this method is quick, it might not be ideal for full-width images, as they would still align to the far left of their container.
The Deprecated <center> Tag
Historically, HTML provided the <center> tag specifically for centering elements. However, it’s crucial to understand why this tag is no longer recommended:
- Separation of Concerns: Modern web development practices advocate for a clear separation between content structure (HTML) and presentation (CSS). The <center> tag blurred this distinction, leading to less maintainable code in the long run.
Margin: auto (for Block-level Images)
This method offers reliable horizontal centering for block-level images. Here’s how it works:
Ensure Block-level Behavior
If your image isn’t already displayed as a block-level element, you can either:
- Wrap it in a block-level container like a <div>.
- Add the CSS property display: block directly to the <img> tag.
Set Automatic Margins
Apply the following CSS to your image:
HTML
img {
display: block; /* Ensure block-level behavior */
margin-left: auto;
margin-right: auto;
}
Explanation:
- By setting display: block, we ensure the image takes up the full width of its container.
- Setting both left and right margins automatically tells the browser to distribute any extra space equally on both sides of the image, effectively centering it horizontally.
This method won’t directly work for vertical centering. Later in the guide, we’ll explore techniques for both horizontal and vertical centering.
Centering Images with Modern CSS Techniques
Flexbox
Flexbox (Flexible Box Layout Module) is a powerful CSS layout mode designed to simplify the arrangement and distribution of elements within containers, even when their sizes are unknown or dynamic. It offers exceptional flexibility for both horizontal and vertical centering.
Basic Flexbox Setup
To use Flexbox, you’ll need a parent container. Apply these CSS properties to it:
CSS
.container {
display: flex; /* Enable Flexbox layout */
}
Let’s break down some essential Flexbox properties:
- display: flex: Activates Flexbox for the container element.
- align-items: center: Aligns items along the vertical axis of the container (for vertical centering).
- justify-content: center: Aligns items along the horizontal axis of the container (for horizontal centering).
Example: Centering an Image with Flexbox
HTML
<div class="container">
<img decoding="async" src="image-url.jpg" alt="Image Description">
</div>
CSS
.container {
display: flex;
align-items: center;
justify-content: center;
}
Elementor simplifies Flexbox usage with its intuitive Flexbox Containers and drag-and-drop interface. Within the Elementor editor, you can easily enable Flexbox layouts and adjust alignment properties effortlessly.
Absolute Positioning with transform: translate(-50%, -50%)
This method provides precise control over an element’s position and is particularly useful for scenarios where you need to center an element within a container that has relative positioning.
Here’s the breakdown:
- Relative Positioning (for the Parent): Ensure the image’s parent container has the CSS property position: relative. This establishes a reference point for the image’s absolute positioning.
- Absolute Positioning (for the Image): Apply the following CSS to your image:
CSS
img {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
Explanation:
- position: absolute removes the image from the normal document flow and allows you to position it precisely using top, left, right, and bottom properties.
- top: 50% and left: 50% position the image’s top-left corner at the center of its container.
- transform: translate(-50%, -50%) is the magic trick! It shifts the image back by 50% of its own width and height, effectively centering it based on its own dimensions.
Note: Make sure the parent container has a defined height and width; otherwise, absolute positioning might have unexpected results.
Vertical Centering Considerations
Unlike horizontal centering, which often has straightforward solutions, achieving perfect vertical image centering within a container can require a combination of techniques. Here’s why:
- Unknown Image Heights: Web pages are dynamic, and images often have varying heights. If the height of the container is fixed, vertical centering is simpler. However, when heights are unknown, the challenge increases.
- Line Heights: Inline elements, like images, are influenced by the concept of line height within their containers. Unexpected line height values can disrupt precise vertical alignment.
Common Techniques
Let’s outline some commonly used methods that leverage the concepts we’ve covered so far:
- Flexbox: As you saw earlier, the `align-items: center` property within a Flexbox container provides a reliable solution for both horizontal and vertical centering.
- Absolute Positioning with a Known Height: If you know the image’s height, you can combine absolute positioning with calculated top margin:
CSS
img {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
margin-top: -25px; /* Half of the image's height */
}
Line-height and display: table-cell (legacy): An older technique involves setting a large `line-height` on a container and using `display: table-cell` with `vertical-align: middle` on the image. While it works, this method is less flexible for modern, responsive layouts.
The most suitable method might depend on your project’s specific structure and requirements.
Responsive Image Centering
In today’s world of multiple screen sizes and devices, ensuring your images look perfect and remain centered across various resolutions is essential. This is where CSS media queries come to the rescue.
Media Queries: The Key to Responsiveness
Media queries enable you to apply different CSS rules based on specific conditions, such as the width of the user’s browser viewport. Here’s a basic example:
CSS
@media (max-width: 768px) {
/* CSS rules for screen widths smaller than 768px */
}
Image Centering Adjustments with Media Queries
Let’s say you want to modify your image centering technique on smaller screens. You might adjust the container’s height or switch from Flexbox to a different method within a media query:
CSS
@media (max-width: 768px) {
.container {
height: 300px; /* Adjust container height as needed */
}
.container img {
margin: auto; /* Use margin: auto for smaller displays */
}
}
Maintaining Image Aspect Ratio with object-fit
While centering your images is important, preventing distortion from stretching or squishing is equally crucial. The CSS object-fit property comes to the rescue:
- object-fit: cover: Scales the image to cover its container while maintaining its aspect ratio. Parts of the image may be clipped.
- object-fit: contain: Scales the image to fit within its container entirely while maintaining its aspect ratio. This might result in some space around the image.
Example:
CSS
img {
width: 100%; /* Image takes up the full container width */
height: auto; /* Maintains aspect ratio */
object-fit: cover; /* Prevents distortion */
}
Centering Images Within Various Page Elements
Often, you’ll want to center images inside specific HTML elements like tables, lists, figures, and other containers. Here’s a breakdown of common scenarios and how to approach them:
Centering Images Within Tables
- For table cells (<td>), apply text-align: center to center the image horizontally. Remember the inline vs. block-level concepts we discussed earlier!
- For vertical centering within table cells, consider using a combination of vertical-align: middle and line-height adjustments.
Centering Images Within Lists
- Target the list item (<li>) containing the image and apply text-align: center. This will center the entire list item’s content, including the image.
Centering Images Within Figures
The <figure> element is often used to group images with captions.
- Wrap both the image and its <figcaption> within a <figure> element.
- Apply text-align: center to the <figure> to center its entire content.
Other Common Containers
For most block-level containers, the techniques we’ve covered (text-align, margin: auto, Flexbox) will generally work well. Here are some additional tips:
Experiment with Combinations: Sometimes, the best solutions involve combining techniques. For instance, using Flexbox on a parent container and then margin: auto on the image within it.
Elementor often provides dedicated widgets or layout options that simplify the process of centering images within various elements. To leverage these tools, explore Elementor’s documentation and visual editor.
Important Note: Always test your image centering across different browsers and screen sizes after making changes to ensure proper rendering.
Image Centering with CSS Grid
CSS Grid is a layout system that excels in creating complex, multi-dimensional grid structures for web pages. It also offers elegant solutions for image centering within grid layouts.
Basic Grid Setup
Here’s how you would set up a basic CSS Grid container:
CSS
.grid-container {
display: grid;
grid-template-columns: repeat(3, 1fr); /* Three equal-width columns */
grid-template-rows: 100px; /* Example row height */
}
Let’s break down the key properties:
- display: grid: Enables CSS Grid layout for the container.
- grid-template-columns: Defines the column structure (here, three columns with equal widths using ‘1fr’ units).
- grid-template-rows: Defines the row structure.
Centering Images within Grid Cells
To center an image within a grid cell, use the following properties on the image itself:
CSS
.grid-container img {
align-items: center;
justify-content: center;
}
Explanation: These properties, similar to their use in Flexbox, instruct the grid to align the image both horizontally and vertically within its designated grid cell.
The Power of CSS Grid
- Multi-dimensional Control: CSS Grid allows you to define and position images within complex rows and columns precisely.
- Flexibility: Easily adjust the number of rows, columns, and their sizes using grid-template-columns and grid-template-rows.
- Responsiveness: Combine CSS Grid with media queries to create adaptive, image-centered layouts that change dynamically based on screen size.
Centering Multiple Images
Often, you’ll encounter scenarios where you need to center a group of images horizontally, vertically, or both. Here’s how to approach this using the methods we’ve discussed:
Horizontal Centering of Multiple Images
Wrapper Element
Wrap your images within a container element like a <div>.
Apply Proven Techniques
- Text-align: Set text-align: center on the wrapper element if your images are inline.
- Flexbox: Use display: flex and justify-content: center on the wrapper.
- CSS Grid: Create a grid layout and use justify-content: center on the container.
Vertical Centering of Multiple Images
Techniques will vary depending on whether you know image heights or have a fixed-height container. Experiment with Flexbox (align-items: center), absolute positioning in combination with transform: translate(), or line-height tricks (if applicable).
Example (Using Flexbox)
HTML
HTML
<div class="image-group">
<img decoding="async" src="image1.jpg" alt="Image 1">
<img decoding="async" src="image2.jpg" alt="Image 2">
<img decoding="async" src="image3.jpg" alt="Image 3">
</div>
CSS
CSS
.image-group {
display: flex;
justify-content: center; /* Horizontal centering */
align-items: center; /* Vertical centering */
}
Note: When centering multiple images, be mindful of responsiveness. Use media queries to adjust the image group’s layout or individual image behavior for different screen sizes.
Centering Background Images
Background images add visual interest and impact to various elements on your website. The CSS background-image and background-position properties are your primary tools for controlling their placement.
Using background image and background-position
Apply the Background Image
Use the background-image property on the element where you want the image displayed:
CSS
.my-element {
background-image: url('image-path.jpg');
}
Center the Image
Utilize the background-position property with the center value:
CSS
.my-element {
background-image: url('image-path.jpg');
background-position: center;
}
Additional Notes
- background-size: Control how the background image scales with properties like cover (scales to cover the entire container) or contain (fits the whole image within the container).
- background-repeat: Use no-repeat to prevent background image repetition.
Background Shorthand
Combine these properties into a single declaration:
CSS
.my-element {
background: url('image-path.jpg') center / cover no-repeat;
}
Best Practices and Optimization for Elementor Websites
Browser Compatibility
While modern browsers generally handle image centering consistently, it’s wise to be mindful of potential inconsistencies in older browsers or those with limited support for newer CSS features.
Cross-Browser Testing
Test your website on different browsers (Chrome, Firefox, Edge, Safari, etc.) and on various devices to ensure your centered images look as intended everywhere.
Vendor Prefixes
In rare cases, consider using vendor prefixes (-webkit-, -moz-, -ms-) for certain CSS properties to maximize compatibility with older browsers.
Progressive Enhancement
Start with basic techniques that work universally, and layer on more advanced features like Flexbox or Grid as enhancements for modern browsers.
Accessibility
Web accessibility is crucial for ensuring your website is usable by everyone, including people with disabilities. Here’s how image-centering ties into accessibility:
- alt Attributes: Always provide descriptive alt text for your images. Screen readers rely on alt text to convey the image’s content to visually impaired users.
- Semantic Structure: Use appropriate heading tags (<h1>, <h2>, etc.), lists, and other HTML elements to give your page a logical structure. This aids both screen readers and search engines in understanding your content.
Elementor Image Optimizer
Image optimization is essential for a fast-loading website. Elementor’s built-in Image Optimizer seamlessly streamlines this process for you:
- Automatic Compression: Elementor’s Image Optimizer can intelligently compress your images to significantly reduce file sizes without compromising visual quality.
- Optimized Image Delivery: Images are automatically resized and served in the most optimal format for the user’s device and browser. This translates to faster loading times and improved user experience.
When to Choose Which Techniques
With so many image-centering options, picking the right one for a particular situation can be daunting. Here’s a decision-making framework to guide you:
- Complexity: For simple horizontal centering, text-align: center or margin: auto is often sufficient. For more intricate layouts or combined horizontal and vertical centering, Flexbox or Grid offers greater flexibility.
- Responsiveness: Consider how the image needs to behave on different screen sizes. Will its container change dimensions? Use media queries and responsive techniques as needed.
- Browser Support: If you need to support very old browsers, stick to the most tried-and-true methods, such as text-align and margin: auto.
To streamline the process, take advantage of Elementor’s intuitive drag-and-drop interface. Elementor often provides Elementor-specific widgets and visual controls for centering images within various elements.
Conclusion
Mastering image centering is an essential skill for creating visually appealing and professional-looking web pages. Whether you’re showcasing products, highlighting testimonials, or simply adding visual flair, understanding the various techniques empowers you to achieve your desired layout outcomes.
Remember these key points:
- HTML and CSS Fundamentals: Image centering relies on a combination of HTML image syntax (<img> tag, alt attribute) and CSS properties like text-align, margin, Flexbox (align-items, justify-content), CSS Grid, and absolute positioning.
- Flexibility: Choose the most appropriate image-centering method based on your project’s complexity, responsiveness needs, and desired level of control.
Elementor streamlines the image-centering process with its drag-and-drop interface, dedicated widgets, and integrated performance optimizations, such as Elementor Image Optimizer and Elementor Hosting powered by Google Cloud and Cloudflare.
By following the best practices outlined in this guide, you’ll be well-equipped to center images confidently within your WordPress website.
Looking for fresh content?
By entering your email, you agree to receive Elementor emails, including marketing emails,
and agree to our Terms & Conditions and Privacy Policy.