Table of Contents
Don’t worry; this guide has everything you need. We’ll break down the fundamental CSS concepts involved in table centering, explore classic and modern techniques, and even delve into some advanced scenarios. By the end, you’ll have a mastery of table alignment and the confidence to create visually balanced web pages, understanding exactly why each method works. So, let’s dive in!
Understanding the Basics
HTML Table Structure Review
Before we delve into CSS, let’s do a quick refresher on the basic HTML tags that make up a table. This will ensure we’re all on the same page, and it’s an opportunity to mention Elementor’s capabilities naturally:
- <table>: The container for the entire table.
- <thead>: Defines the table’s header row(s).
- <tbody>: Contains the main table body data.
- <tr>: Represents a single table row.
- <th>: A header cell (usually bold and centered by default).
- <td>: A standard data cell.
If you’re building your website with Elementor, you can easily create and customize tables visually using the Table widget. Elementor handles the underlying HTML structure for you!
The Core CSS Concepts
Now, let’s break down the key CSS properties that are essential for understanding table centering:
- margin: Controls the space around an element. Setting margin-left and margin-right to auto is a classic way to center block-level elements, including tables horizontally.
- display: Dictates how an element is rendered in the browser. The default for tables is display: table; Changing this to display: block; enables certain centering techniques.
- text-align: Primarily aligns text content within an element. While it can center text inside table cells, it doesn’t always center the table itself (we’ll explore this distinction later).
- width & max-width: Control the overall width of a table. These properties play a crucial role in how some centering methods function, and they are essential for ensuring your tables look great on different screen sizes.
Grow Your Sales
- Incredibly Fast Store
- Sales Optimization
- Enterprise-Grade Security
- 24/7 Expert Service
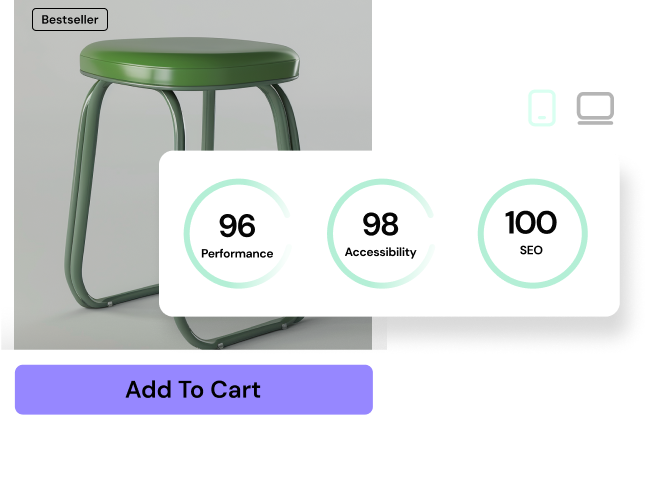
- Incredibly Fast Store
- Sales Optimization
- Enterprise-Grade Security
- 24/7 Expert Service
- Prompt your Code & Add Custom Code, HTML, or CSS with ease
- Generate or edit with AI for Tailored Images
- Use Copilot for predictive stylized container layouts
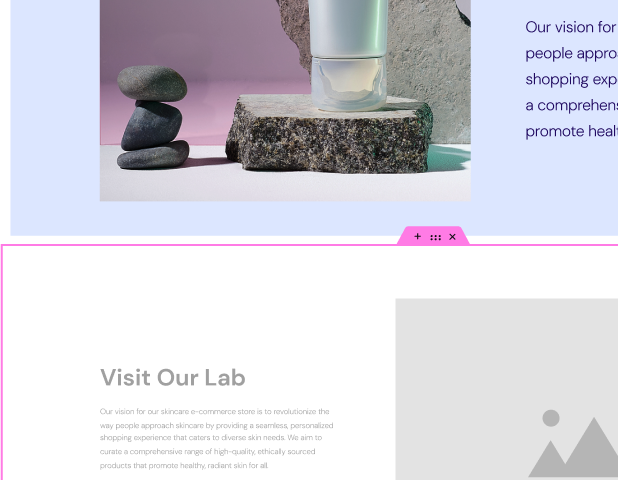
- Prompt your Code & Add Custom Code, HTML, or CSS with ease
- Generate or edit with AI for Tailored Images
- Use Copilot for predictive stylized container layouts
- Craft or Translate Content at Lightning Speed
Top-Performing Website
- Super-Fast Websites
- Enterprise-Grade Security
- Any Site, Every Business
- 24/7 Expert Service
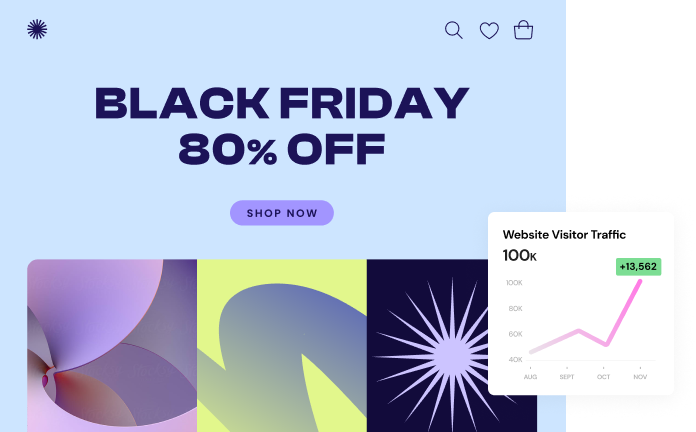
Top-Performing Website
- Super-Fast Websites
- Enterprise-Grade Security
- Any Site, Every Business
- 24/7 Expert Service
- Drag & Drop Website Builder, No Code Required
- Over 100 Widgets, for Every Purpose
- Professional Design Features for Pixel Perfect Design
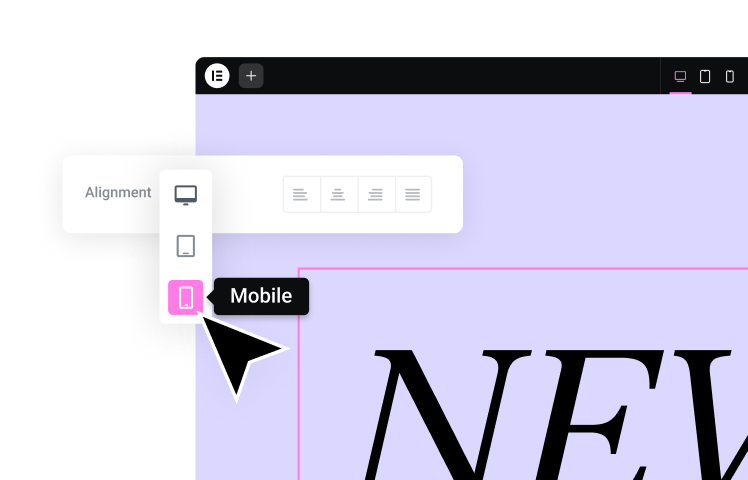
- Drag & Drop Website Builder, No Code Required
- Over 100 Widgets, for Every Purpose
- Professional Design Features for Pixel Perfect Design
- Marketing & eCommerce Features to Increase Conversion
- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking
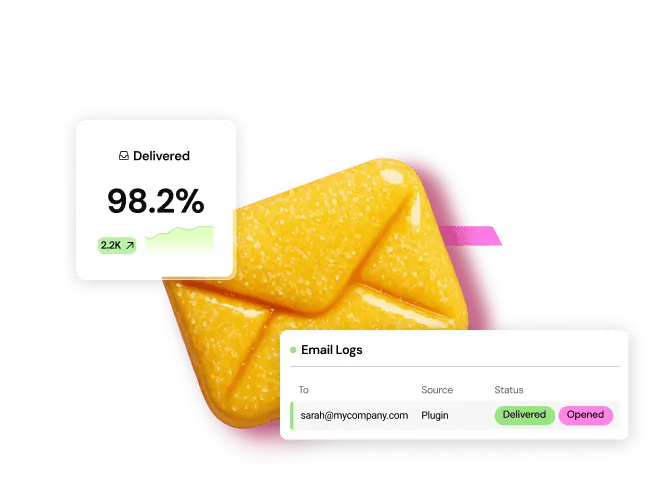
- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking
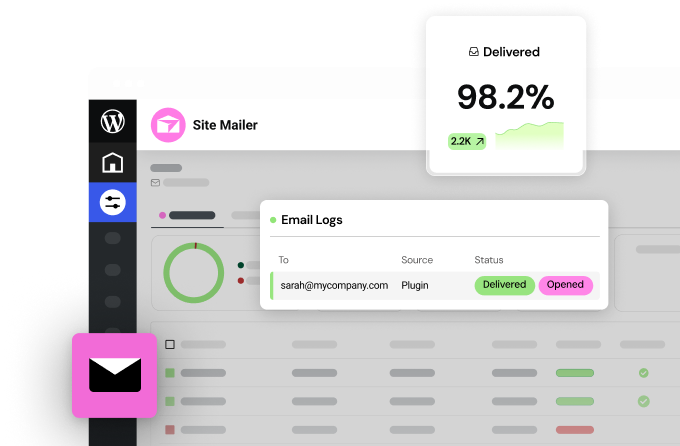
- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking
The Classic Methods
Method 1: margin: 0 auto;
This is the most well-known approach to centering a table (and many other block-level elements). Here’s how it works:
- Set Margins to Auto: By applying margin-left: auto; and margin-right: auto; to your table, you’re telling the browser to automatically distribute any leftover space equally on both sides, effectively pushing the table into the center.
- Width Considerations: This method generally works best when your table’s width is smaller than its container. If the table is set to width: 100%, it will already take up all the horizontal space, leaving no room for automatic margins to work their magic.
Example CSS Code:
CSS
table {
margin-left: auto;
margin-right: auto;
/* Optional: Set a width if needed */
width: 80%;
}
Advantages
- Simple and intuitive to understand.
- Widely supported across different browsers.
Limitations
- Requires the table’s width to be less than its container.
- It can sometimes behave unexpectedly in complex layouts.
Let’s move on to the next classic method!
Method 2: display: block;
Here’s the breakdown of this technique:
- Change the Display Type: By setting display: block; on your table, you transform it into a block-level element. This change in behavior makes it respond to margins in the same way that other common block elements (like <div> or <p>) do.
- Combine with Automatic Margins: Now that your table is a block element, you can use the margin: 0 auto; technique to achieve horizontal centering.
Example CSS Code:
CSS
table {
display: block;
margin-left: auto;
margin-right: auto;
}
Advantages
- Straightforward and reliable.
Limitations
- It may have unintended side effects if you rely on the default table-specific layout behaviors elsewhere in your design.
Method 3: text-align: center;
This method often trips people up because it sounds like it should center the table itself. Here’s the crucial distinction to remember:
- text-align: center; centers the content within elements, not the element itself. For tables, this means it will center the text inside your table cells (<th> and <td>) but won’t necessarily move the entire table to the center of its container.
When is this useful?
- In Combination: Sometimes, you might use text-align: center; on the table cells in conjunction with one of the other centering methods (like margin: 0 auto;) to achieve both table centering and cell content centering.
- Content-Specific Alignment: If your primary goal is to ensure text within all your table cells is center-aligned, regardless of the table’s position, then text-align: center; is the way to go.
Example CSS Code:
CSS
table {
/* Some other centering method (e.g., margin: 0 auto;) */
}
th, td {
text-align: center;
}
If you’re using Elementor, you often have visual controls within the Table widget to adjust the alignment of text within cells independently of how the table itself is positioned.
Responsive & Modern Approaches
Centering Tables & Responsiveness
Picture this: You’ve carefully centered a beautiful table on your desktop screen. But when you view your website on your phone, the table either spills off the screen or becomes squished and unreadable. Frustrating, right? Responsive design is here to save the day!
The challenge with tables is that they have a naturally rigid structure. Here’s how we ensure they look great on all screen sizes:
- width and max-width: Using percentage-based widths or setting a max-width: 100%; allows your table to scale down gracefully on smaller screens.
- Mobile-First Thinking: Start by designing for smaller screens and progressively add styles for larger displays. This helps prevent layout issues from cropping up on mobile devices.
- Overflow Handling: If your table has many columns, consider using overflow-x: auto to introduce horizontal scrolling on smaller screens and maintain readability.
Example CSS:
CSS
table {
max-width: 100%;
overflow-x: auto;
}
Flexbox to the Rescue: justify-content: center;
Flexbox is a modern CSS layout module that offers incredible flexibility (hence the name!). Here’s how it streamlines table centering:
- The Flex Container: You’ll need to wrap your table in a container element (usually a <div>). Apply display: flex; to this container to turn it into a flex container.
- The Magic Property: Set justify-content: center; on the flex container. This tells the browser to center all its direct children (in our case, the table) horizontally.
Example CSS:
HTML
<div class="table-container">
<table>
{/* Your table content */}
</table>
</div>
CSS
.table-container {
display: flex;
justify-content: center;
}
Bonus – Flexbox also gives you easy vertical centering with align-items: center;. Explore using Flexbox for your overall website layout – it’s a powerful tool! (If you have an existing Elementor resource on Flexbox, this is a great place to link to it)
The Power of CSS Grid: grid-template-columns & justify-items
CSS Grid is another fantastic layout tool, particularly well-suited for creating complex, multi-dimensional layouts. Here’s how to use it for table centering:
- The Grid Container: Similar to Flexbox, you’ll wrap your table in a container. Apply display: grid; to this container.
- Flexible Column Setup: With grid-template-columns, you can define the number of columns your grid should have. A simple grid-template-columns: auto will often do the trick, creating a single column that takes up the available space.
- Centering with Ease: Now, use justify-items: center; on the grid container. This tells the browser to center all direct children horizontally within their grid cell.
Example CSS:
HTML
<div class="table-container">
<table>
{/* Your table content */}
</table>
</div>
CSS
CSS
.table-container {
display: grid;
grid-template-columns: auto;
justify-items: center;
}
Why Choose Grid?
- Greater Layout Control: Grid excels at situations where you need precise control over both rows and columns.
- Complex Tables: If you have tables with intricate structures, CSS Grid provides the tools to position elements exactly where you want them.
Note: If you’re building with Elementor, check out their resources for using CSS Grid. This is a great place to introduce Elementor’s capabilities without being overly promotional.
Advanced Considerations
Absolute Positioning Techniques
When standard centering methods don’t quite cut it, absolute positioning can offer more fine-grained control. Here’s the basic idea:
- Relative Parent: The table’s container element needs to have a position: relative; This creates a reference point for our absolute positioning.
- Absolute Child: Set the table itself to position absolute. This removes it from the normal document flow.
- Positioning: Use top: 50%; and left: 50%; to place the table’s center at the center of its container.
- Negative Margins: Employ negative margins (margin-top and margin-left set to half the table’s dimensions) to shift the table back, ensuring it’s perfectly centered.
Example CSS:
HTML
<div class="table-container">
<table>
{/* Your table content */}
</table>
</div>
CSS
.table-container {
position: relative;
}
table {
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
Important: The transform: translate(-50%, -50%); technique is more modern and reliable for precise centering of absolutely positioned elements.
When to Use This
- Specific Layout Needs: When you need to place a table in a very precise location, regardless of surrounding content.
- Overlays: Creating overlays or modal popups where the table should be centered within the viewport.
Let’s move on to potential browser compatibility quirks!
Browser Quirks & Troubleshooting
While modern browsers are remarkably consistent with CSS table styling, there are some things to keep in mind:
Older Browsers
If you need to support very old versions of Internet Explorer (IE), some techniques might behave unexpectedly. Consider fallbacks or conditional styles for these cases.
Debugging Tips
If your centering doesn’t work, here’s your checklist:
- Check for Conflicting Styles: Make sure other CSS rules aren’t overriding your centering styles.
- Inspect Element: Use your browser’s developer tools to see the applied styles and computed dimensions of the table and its container.
- Compatibility Testing: Test your website in different browsers (Chrome, Firefox, Edge, etc.) and on various devices.
Elementor’s visual builder and debugging tools can often streamline this troubleshooting process, hinting at the advantages of the platform.
When NOT to Center Tables
Web design isn’t just about technical tricks; it’s about creating visually pleasing and intuitive layouts. There are times when a left-aligned table actually enhances the user experience:
- Data-Heavy Tables: If your table contains lots of text-based information, left-alignment often improves readability, as our eyes naturally scan from left to right.
- Consistency: Maintain a consistent alignment scheme within a specific webpage or section to avoid a visually jarring layout.
- Narrow Columns: Centering tables with very narrow columns can sometimes make them appear awkward or create unbalanced whitespace.
- Aesthetic Choices: Sometimes, a left-aligned table simply integrates better with your website’s overall design, contributing to a clean, modern look.
Design Tip: Don’t be afraid to experiment! Try both centered and left-aligned versions of your table and see which one harmonizes best with your website’s aesthetic and content structure.
Elementor & Optimized Centering
Elementor’s Design Advantages
Elementor’s visual builder and intuitive Table widget bring several key benefits to table creation and centering:
- No-Code Centering: Drag and drop your table into place and use Elementor’s visual alignment controls to achieve perfect centering with just a few clicks.
- Responsive Refinement: Elementor’s responsive editing tools make this process easy. You can easily adjust how your table behaves on different screen sizes, ensuring it looks fantastic on desktops, tablets, and phones.
- Customization Galore: Style your tables to match your brand perfectly. Tweak fonts, colors, cell spacing, borders, and more – all without writing complex CSS.
If any specific Elementor styling options directly relate to table centering, call them out!
Conclusion
By now, you’ve mastered the art of centering tables in CSS! Whether you’re using classic margin techniques, the power of Flexbox and Grid, or even some advanced absolute positioning tricks, you have the tools to create perfectly aligned tables for any web design project.
Remember, the best centering method often depends on several factors:
- Table complexity: Simple tables only need a margin of 0 auto, while more intricate structures could benefit from Grid.
- Responsiveness: Ensure your tables gracefully adapt to different screen sizes using techniques such as width, max width, and potentially overflow.
- Design Context: Sometimes, a left-aligned table might be a more aesthetically pleasing choice.
If you’re using Elementor, the process becomes even more intuitive, thanks to its visual builder, responsive controls, and optimized hosting environment.
Looking for fresh content?
By entering your email, you agree to receive Elementor emails, including marketing emails,
and agree to our Terms & Conditions and Privacy Policy.