Table of Contents
In this comprehensive guide, we’ll dive into how to add CSS to your HTML code. We’ll cover the three main methods (inline, internal, and external), explore essential CSS properties, and share how a powerful website builder like Elementor, with its integrated hosting, can streamline your CSS workflow. Whether you’re a beginner or ready to level up, this article aims to be your ultimate CSS companion. So, let’s get styling!
Understanding CSS Basics
CSS Syntax
CSS might look strange at first, but its underlying structure is quite simple. Let’s break it down:
- Selector: Targets the HTML element(s) you want to style (e.g., h1, p, .my-class).
- Property: The style aspect you want to change (e.g., color, font-size, background-image).
- Value: The specific setting for the property (e.g., red, 16px, url(‘background.jpg’)).
A Basic Example
CSS
p { /* Selector */
color: blue; /* Property: value */
font-family: Arial, sans-serif; /* Property: value */
}
This code would make all paragraph (<p>) elements blue with an Arial font (or a generic sans-serif font as a fallback).
The Cascade
CSS, as the name implies, works in a cascading manner. This means multiple styles can apply to the same element, and the browser determines the final look based on a hierarchy. The most specific selector usually wins out.
Specificity
How does the browser decide which rule is most specific? There’s a scoring system:
- Inline styles (highest priority)
- ID selectors
- Class, attribute, and pseudo-class selectors
- Element selectors
- Universal selector (*)
Inheritance
Some CSS properties, like color and font-family, are inherited. This means if you style a parent element (like <body>), its child elements (like paragraphs and headings within it) can inherit those styles unless specifically overridden.
Grow Your Sales
- Incredibly Fast Store
- Sales Optimization
- Enterprise-Grade Security
- 24/7 Expert Service
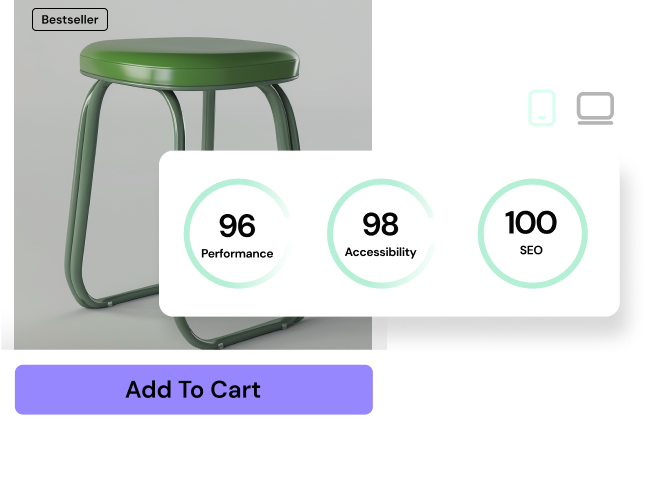
- Incredibly Fast Store
- Sales Optimization
- Enterprise-Grade Security
- 24/7 Expert Service
- Prompt your Code & Add Custom Code, HTML, or CSS with ease
- Generate or edit with AI for Tailored Images
- Use Copilot for predictive stylized container layouts

- Prompt your Code & Add Custom Code, HTML, or CSS with ease
- Generate or edit with AI for Tailored Images
- Use Copilot for predictive stylized container layouts
- Craft or Translate Content at Lightning Speed
Top-Performing Website
- Super-Fast Websites
- Enterprise-Grade Security
- Any Site, Every Business
- 24/7 Expert Service

Top-Performing Website
- Super-Fast Websites
- Enterprise-Grade Security
- Any Site, Every Business
- 24/7 Expert Service
- Drag & Drop Website Builder, No Code Required
- Over 100 Widgets, for Every Purpose
- Professional Design Features for Pixel Perfect Design
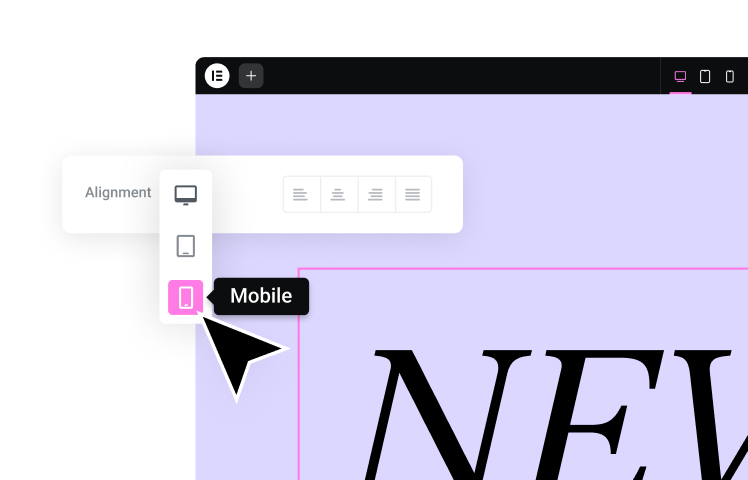
- Drag & Drop Website Builder, No Code Required
- Over 100 Widgets, for Every Purpose
- Professional Design Features for Pixel Perfect Design
- Marketing & eCommerce Features to Increase Conversion
- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking

- Ensure Reliable Email Delivery for Your Website
- Simple Setup, No SMTP Configuration Needed
- Centralized Email Insights for Better Tracking
Methods for Adding CSS to HTML
There are three primary ways to integrate CSS into your HTML documents:
1. Inline CSS
Syntax and Examples
Inline CSS involves adding the style attribute directly within an HTML element. Here’s an example:
HTML
<p style="color: red; font-size: 20px;">This is a red paragraph.</p>
Use Cases
Inline CSS is best suited for quick, one-off changes to individual elements. It’s helpful for testing or making a single element unique.
Pros and Cons
- Pros: Direct, hyper-specific styling takes the highest priority in the cascade.
- The cons are that it clutters HTML, is difficult to maintain, and is not scalable for large websites. If used excessively, it can lead to repetitive code and make updates tedious.
2. Internal CSS
The <style> element: Internal CSS uses the <style> tag placed within the <head> section of your HTML document.
HTML
<head>
<style>
body {
background-color: lightblue;
}
h1 {
color: navy;
text-align: center;
}
</style>
</head>
Scope
Styles defined within <style> apply to the entire HTML document.
Pros and Cons
- Pros: Improves organization compared to inline CSS, suitable for styling a single page.
- Cons: Cannot be reused across multiple HTML files, making site-wide changes more cumbersome.
While internal CSS is a step up from inline, using a website builder like Elementor’s theme builder offers centralized control for managing site-wide styles, templates, and reusable components.
3. External CSS
The <link> Element: External CSS involves creating a separate .css file and referencing it within your HTML’s <head> using the <link> tag. Here’s how it works:
HTML
<head>
<link rel="stylesheet" type="text/css" href="styles.css">
</head>
Important attributes
- rel=”stylesheet”: Specifies the relationship between the HTML and the linked file.
- type=”text/css”: Defines the content type of the linked file.
- href=”styles.css”: The path to your CSS file.
File Structure and Organization
For a clean project structure, it’s best practice to keep your CSS files in a dedicated folder (e.g., /css/styles.css).
Pros and Cons
- Pros: Ultimate separation of concerns (HTML for structure, CSS for styling), promotes code reusability across multiple HTML pages, browser caching (faster loading on subsequent visits), and is indispensable for large, multi-page websites.
- Cons: There is slight overhead on initial page load (the extra request to fetch the CSS file).
External CSS is the recommended approach for most web projects due to its maintainability, scalability, and performance benefits.
Practical CSS Properties for Customization
Now that you understand the ways to add CSS, let’s explore some of the most common and useful properties you’ll use to style your website.
Colors and Backgrounds
Color Formats: You can define colors in various ways:
- Hex codes: e.g., #FF0000 (red), #008000 (green)
- RGB: e.g., rgb(255, 0, 0) (red)
- RGBA: Adds an alpha channel (transparency) e.g., rgba(0, 0, 0, 0.5) (semi-transparent black)
- Named Colors: e.g., blue, orange, yellowgreen
background-color: Sets the background color of an element:
CSS
div {
background-color: lightgray;
}
background-image: This lets you use an image as a background:
CSS
body {
background-image: url('pattern.jpg');
}
Gradients: You can even create beautiful gradients using CSS:
CSS
.banner {
background: linear-gradient(to right, red, orange);
}
Text Styling
font-family: Specifies the font(s) to use:
CSS
p {
font-family: Arial, Helvetica, sans-serif;
}
font-size: Controls the size of the text (common units include pixels px, percentages %, and responsive units like em):
CSS
h1 {
font-size: 36px;
}
font-weight: Sets how bold or thin the text appears (normal, bold, lighter, numerical values 100-900):
CSS
p {
font-weight: normal;
}
strong {
font-weight: bold;
}
Spacing and Layout
margin: Controls the space outside an element’s border. You can set margins for all sides at once or individual sides (top, right, bottom, left):
CSS
p {
margin: 20px; /* Creates a 20px margin on all sides */
margin-bottom: 30px; /* Adds extra space below */
}
padding: Controls the space between an element’s content and its border. Similar to margin, it can be applied to all sides or individually:
CSS
.content-box {
padding: 15px;
}
The CSS Box Model
It’s crucial to understand that every element in HTML is essentially a box. The box model consists of:
- Content: The actual text or image within the element.
- Padding: The transparent area around the content.
- Border: The visible border surrounding the padding (can be styled with border-width, border-style, border-color).
- Margin: Transparent area outside the border.
Width and height: These properties control the dimensions of an element’s content area:
CSS
img {
width: 100%; /* Makes images responsive */
max-width: 500px; /* Prevents images from being too large */
}
Display and Positioning
Display
This fundamental property controls how an element behaves within the layout:
- block: Elements take up the full width available, start on a new line (e.g., headings, paragraphs, divs).
- inline: Elements take up only as much space as necessary, so don’t start on a new line (e.g., links or spans within text).
- inline-block: A hybrid, allowing you to set the width and height of an inline element.
- none: The element is completely hidden.
Position
Fine-tunes the placement of elements:
- static: Default behavior.
- relative: Used for positioning relative to its normal flow, often used in conjunction with top, bottom, left, and right.
- absolute: Positions an element relative to its nearest positioned ancestor (or the body if none exists). Removed from normal flow.
- fixed: Positions an element relative to the browser viewport (e.g., a sticky menu).
Advanced CSS Techniques
Responsive Design
In today’s world of multiple devices, making your website look great on desktops, tablets, and phones is non-negotiable. That’s where responsive design comes in.
Media Queries: The backbone of responsiveness, media queries let you apply different CSS rules based on screen size, orientation, and other device features. Here’s an example:
CSS
@media (max-width: 768px) {
/* Styles for smaller screens */
.content {
width: 100%;
}
}
CSS Frameworks
- Brief Overview: CSS frameworks like Bootstrap, Tailwind CSS, and others provide pre-built components (buttons, grids, navigation, etc.) and utility classes to speed up development.
- Subtle comparison: While frameworks offer convenience, using a visual tool like Elementor gives you the utmost flexibility when it comes to crafting truly unique designs without being overly reliant on framework-specific structures.
CSS Preprocessors
- Sass, Less: Preprocessors extend CSS with features like variables, nesting, mixins (reusable blocks of code), and more, making your stylesheets more organized and efficient.
Common CSS Issues and Fixes
- Conflicting Styles: Ensure the cascade and specificity are working as intended. Use developer tools to pinpoint the source of conflicts.
- Cross-browser Incompatibilities: Test your website in different browsers. Use prefixes for experimental CSS properties as needed.
- Layout Problems: Become familiar with the box model and tools like display, position, floats (clear), and newer layout techniques like Flexbox and CSS Grid.
CSS Validation
Use a CSS validator to ensure your code follows CSS standards. This helps catch potential errors.Best Practices
- Organization: Use comments and a logical file structure.
- Naming Conventions: Adopt consistent naming for classes and IDs (e.g., BEM methodology).
- .Avoid Excessive Nesting: This can make your CSS harder to read and maintain.
- Prioritize Maintainability: Write CSS with future changes in mind.
The Power of Elementor Website Builder
While this guide has equipped you with a solid CSS foundation, let’s explore how Elementor Website Builder and its integrated hosting solution can make your CSS journey smoother and more efficient.Seamless Integration
- Visual Styling: Elementor’s drag-and-drop interface lets you adjust colors, fonts, spacing, and more with just a few clicks. You can also see your CSS changes happen in real time.
- Global Styling: Define site-wide styles through Elementor’s theme builder, ensuring consistency without repetitive CSS across pages.
- Responsive Controls: Precisely adjust how elements look on different devices using Elementor’s responsive editing mode.
Conclusion
CSS is the language that unlocks the web’s visual potential. By understanding its core concepts, techniques, and best practices, you can create stunning and engaging websites. Whether you’re a beginner or seasoned with CSS, a powerful website builder like Elementor, paired with its integrated hosting, can amplify your results. It helps you implement CSS more intuitively, manage styles with ease, and benefit from performance optimizations that keep your website loading fast.Additional Tips for Future Growth
- Stay Curious: CSS and web standards are always evolving. Subscribe to web design blogs, follow CSS experts, and keep yourself updated on new techniques and features.
- Experiment: The best way to learn is by doing and trying different things. Don’t be afraid to tinker with code and see how changes impact the visual presentation.
- Community Resources: Engage in online forums and communities for web developers. Get answers to your questions, share your work, and learn from others.
Looking for fresh content?
By entering your email, you agree to receive Elementor emails, including marketing emails,
and agree to our Terms & Conditions and Privacy Policy.